public static String getClassName(Class clazz){ return clazz.getName(); } /** * 获取Entity注解中的表名 */ public static String getTableName(Class clazz){ if (clazz.isAnnotationPresent(Entity.class)){ Entity entity = (Entity) clazz.getAnnotation(Entity.class); return entity.name(); } else { System.out.println("缺少Entity注解"); return null; } } /** * 获取主键属性和对应的字段 */ public static Map<String, String> getIdMapper(Class clazz){ boolean flag=true; Map<String, String> map = new HashMap<>(); Field[] fields = clazz.getDeclaredFields(); for (Field field:fields){ if (field.isAnnotationPresent(Id.class)){ flag=false; String fieldName = field.getName(); if (field.isAnnotationPresent(Column.class)){ Column column = field.getAnnotation(Column.class); String columnName = column.name(); map.put(fieldName,columnName); break; } else { System.out.println("缺少Column注解"); } } } if (flag){ System.out.println("缺少Id注解"); } return map; }
时间: 2024-02-10 18:19:15 浏览: 78
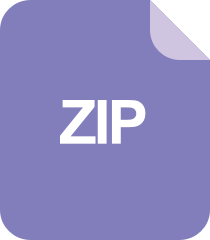
vb.net利用FindWindowEx 枚举窗口句柄,并向句柄发送字符,模拟按键。

这段代码是用来获取实体类对应的表名和主键属性以及对应的字段名的。其中,getClassName方法用来获取类的全限定名,getTableName方法用来获取实体类对应的表名,getIdMapper方法用来获取主键属性和对应的字段名。这些方法都是静态方法,可以直接通过类名调用。该代码使用了Java反射机制,通过获取类的注解和属性上的注解来获取需要的信息。如果类或属性上缺少必要的注解,则会输出相应的错误信息。
阅读全文
相关推荐
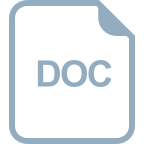
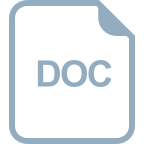

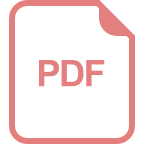













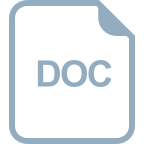