汽车销售租赁管理平台Java代码
时间: 2024-01-28 16:04:18 浏览: 18
汽车销售租赁管理平台涉及到多个模块,包含前端和后端。以下是一个简单的Java代码示例,实现了一个汽车租赁模块的后端代码:
```java
package com.example.carrental.demo.controller;
import com.example.carrental.demo.entity.Car;
import com.example.carrental.demo.entity.RentalRecord;
import com.example.carrental.demo.service.CarService;
import com.example.carrental.demo.service.RentalRecordService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.Date;
import java.util.List;
@RestController
@RequestMapping("/rental")
public class RentalRecordController {
@Autowired
private RentalRecordService rentalRecordService;
@Autowired
private CarService carService;
@GetMapping("/getAllRentalRecords")
public List<RentalRecord> getAllRentalRecords() {
return rentalRecordService.getAllRentalRecords();
}
@PostMapping("/addRentalRecord")
public void addRentalRecord(@RequestBody RentalRecord rentalRecord) {
rentalRecordService.addRentalRecord(rentalRecord);
Car car = carService.getCarById(rentalRecord.getCarId());
car.setIsRented(true);
carService.updateCar(car);
}
@PostMapping("/returnCar")
public void returnCar(@RequestParam int rentalRecordId) {
RentalRecord rentalRecord = rentalRecordService.getRentalRecordById(rentalRecordId);
rentalRecord.setReturnDate(new Date());
int days = (int) ((rentalRecord.getReturnDate().getTime() - rentalRecord.getRentalDate().getTime()) / (1000 * 3600 * 24));
Car car = carService.getCarById(rentalRecord.getCarId());
car.setIsRented(false);
car.setMileage(car.getMileage() + rentalRecord.getDistance());
carService.updateCar(car);
rentalRecord.setRentalFee(days * car.getDailyRentalFee());
rentalRecordService.updateRentalRecord(rentalRecord);
}
}
```
该代码中,使用了Spring Boot框架,实现了一个租赁记录的控制器类。包含了获取所有租赁记录、添加租赁记录、归还车辆等方法。具体实现过程中,调用了租赁记录服务类和汽车服务类,实现对租赁记录和汽车信息的增删改查操作。
相关推荐
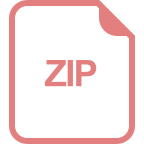
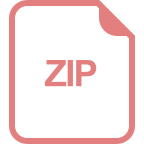
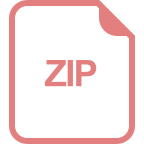














