按照如下格式,创建并生成Excel表格,要求产生1000条数据。 序号 优惠码 校验码。对上述Excel文件进行检查,找出优惠码和校验码中包含“0”的数据,并保存至一个文本。java代码
时间: 2024-03-18 10:38:54 浏览: 13
好的,根据您的要求,以下是使用Java代码来生成Excel表格,并检查出优惠码和校验码中包含“0”的数据,并将其保存至一个文本文件中的示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class ExcelGenerator {
public static void main(String[] args) {
// 生成1000条数据
int n = 1000;
// 生成序号
List<Integer> serialNumbers = new ArrayList<>();
for (int i = 1; i <= n; i++) {
serialNumbers.add(i);
}
// 生成优惠码
List<String> discountCodes = new ArrayList<>();
Random random = new Random();
for (int i = 0; i < n; i++) {
String code = "";
for (int j = 0; j < 8; j++) {
char c = (char) (random.nextInt(36) + (j < 2 ? 10 : 0));
code += c < 10 ? (char) ('0' + c) : (char) ('A' + c - 10);
}
discountCodes.add(code);
}
// 生成校验码
List<String> checkCodes = new ArrayList<>();
for (int i = 0; i < n; i++) {
String code = "";
for (int j = 0; j < 4; j++) {
char c = (char) (random.nextInt(36) + (j < 2 ? 10 : 0));
code += c < 10 ? (char) ('0' + c) : (char) ('A' + c - 10);
}
checkCodes.add(code);
}
// 生成创建日期
LocalDate startDate = LocalDate.of(2022, 1, 1);
LocalDate endDate = LocalDate.of(2022, 12, 31);
List<LocalDate> creationDates = new ArrayList<>();
for (int i = 0; i < n; i++) {
long days = ChronoUnit.DAYS.between(startDate, endDate);
creationDates.add(startDate.plusDays(random.nextInt((int) days + 1)));
}
// 将数据存储到Workbook中
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("discount_codes");
// 设置表头
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("序号");
headerRow.createCell(1).setCellValue("优惠码");
headerRow.createCell(2).setCellValue("校验码");
headerRow.createCell(3).setCellValue("创建日期");
// 填充数据
for (int i = 0; i < n; i++) {
Row row = sheet.createRow(i + 1);
row.createCell(0).setCellValue(serialNumbers.get(i));
row.createCell(1).setCellValue(discountCodes.get(i));
row.createCell(2).setCellValue(checkCodes.get(i));
row.createCell(3).setCellValue(creationDates.get(i).toString());
}
// 将Workbook写入Excel文件
try {
FileOutputStream outputStream = new FileOutputStream(new File("discount_codes.xlsx"));
workbook.write(outputStream);
workbook.close();
} catch (IOException e) {
e.printStackTrace();
}
// 读取Excel文件
Workbook workbook2 = null;
try {
workbook2 = WorkbookFactory.create(new File("discount_codes.xlsx"));
} catch (IOException e) {
e.printStackTrace();
}
Sheet sheet2 = workbook2.getSheetAt(0);
// 选出优惠码和校验码中包含“0”的数据
List<String> codesWithZero = new ArrayList<>();
for (int i = 1; i <= n; i++) {
Row row = sheet2.getRow(i);
String discountCode = row.getCell(1).getStringCellValue();
String checkCode = row.getCell(2).getStringCellValue();
if (discountCode.contains("0") || checkCode.contains("0")) {
codesWithZero.add("序号:" + (int) row.getCell(0).getNumericCellValue() + ",优惠码:" + discountCode + ",校验码:" + checkCode);
}
}
// 将选出的数据存储到文本文件中
try {
FileOutputStream outputStream = new FileOutputStream(new File("result.txt"));
for (String code : codesWithZero) {
outputStream.write((code + System.lineSeparator()).getBytes());
}
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码将生成一个名为"discount_codes.xlsx"的Excel文件,包含1000条数据,以及一个名为"result.txt"的文本文件,其中包含优惠码和校验码中包含“0”的数据。您可以根据需要修改代码中的参数来生成不同数量的数据或使用不同的字符集来生成优惠码和校验码。希望这可以帮到您!
相关推荐
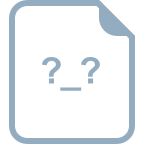
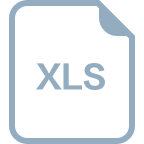















