请用c++帮我写出使用sting字符串来实现CSing中的 Replace,Trim,ComprewNOcase,Left,Right,FInd等方法
时间: 2024-03-02 10:49:56 浏览: 66
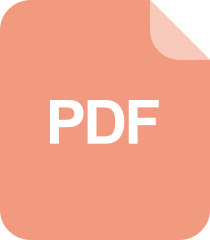
C++中sting类的简单实现方法
好的,下面是使用sting字符串来实现CSing中的 Replace,Trim,ComprewNOcase,Left,Right,Find等方法的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
// Replace 方法
void Replace(string& str, const string& oldStr, const string& newStr)
{
size_t pos = 0;
while ((pos = str.find(oldStr, pos)) != string::npos)
{
str.replace(pos, oldStr.length(), newStr);
pos += newStr.length();
}
}
// Trim 方法
void Trim(string& str)
{
size_t startPos = str.find_first_not_of(" \t\r\n");
if (startPos != string::npos)
{
str.erase(0, startPos);
size_t endPos = str.find_last_not_of(" \t\r\n");
if (endPos != string::npos)
{
str.erase(endPos + 1);
}
}
else
{
str.clear();
}
}
// CompareNOcase 方法
bool CompareNOcase(const string& str1, const string& str2)
{
if (str1.length() != str2.length())
{
return false;
}
for (size_t i = 0; i < str1.length(); ++i)
{
if (tolower(str1[i]) != tolower(str2[i]))
{
return false;
}
}
return true;
}
// Left 方法
string Left(const string& str, size_t count)
{
return str.substr(0, count);
}
// Right 方法
string Right(const string& str, size_t count)
{
return str.substr(str.length() - count, count);
}
// Find 方法
size_t Find(const string& str, const string& subStr)
{
return str.find(subStr);
}
int main()
{
// 测试 Replace 方法
string testStr1 = "Hello, World!";
Replace(testStr1, "World", "C++");
cout << testStr1 << endl;
// 测试 Trim 方法
string testStr2 = " \t\r\n Hello, World! \t\r\n ";
Trim(testStr2);
cout << testStr2 << endl;
// 测试 CompareNOcase 方法
string testStr3 = "Hello, World!";
string testStr4 = "hElLo, wOrLd!";
bool isSame = CompareNOcase(testStr3, testStr4);
cout << (isSame ? "Same" : "Different") << endl;
// 测试 Left 方法
string testStr5 = "Hello, World!";
string leftStr = Left(testStr5, 5);
cout << leftStr << endl;
// 测试 Right 方法
string testStr6 = "Hello, World!";
string rightStr = Right(testStr6, 6);
cout << rightStr << endl;
// 测试 Find 方法
string testStr7 = "Hello, World!";
size_t pos = Find(testStr7, "World");
cout << pos << endl;
return 0;
}
```
希望能够帮到你!
阅读全文
相关推荐
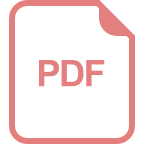
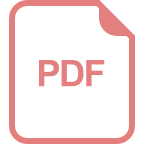















