使用boost库写一个简单状态机的例子
时间: 2023-09-09 17:05:48 浏览: 47
好的,这里是一个使用boost库写的简单状态机的例子:
```c++
#include <iostream>
#include <boost/msm/back/state_machine.hpp>
using namespace std;
namespace msm = boost::msm;
namespace mpl = boost::mpl;
// 定义状态机事件
struct event1 {};
struct event2 {};
struct event3 {};
// 定义状态机状态
struct state1 : msm::front::state<> {};
struct state2 : msm::front::state<> {};
struct state3 : msm::front::state<> {};
// 定义状态机转换规则
struct transition_table : mpl::vector<
msm::front::Row<state1, event1, state2>,
msm::front::Row<state2, event2, state3>,
msm::front::Row<state3, event3, state1>
> {};
// 定义状态机
typedef msm::back::state_machine<
transition_table
> state_machine;
// 定义状态机执行函数
void run_state_machine(state_machine& sm)
{
sm.start(); // 启动状态机
// 发送事件,执行状态转换
sm.process_event(event1());
sm.process_event(event2());
sm.process_event(event3());
sm.stop(); // 停止状态机
}
int main()
{
state_machine sm;
run_state_machine(sm);
return 0;
}
```
这个状态机有三个状态:state1、state2、state3,它们之间可以根据事件 event1、event2、event3 进行转换。在 main 函数中,我们首先创建了一个 state_machine 对象,然后调用 run_state_machine 函数来执行状态机。在 run_state_machine 函数中,我们启动状态机,然后依次发送 event1、event2、event3 事件,最后停止状态机。
当执行到 sm.process_event(event1()); 时,状态机会从 state1 转换到 state2;当执行到 sm.process_event(event2()); 时,状态机会从 state2 转换到 state3;当执行到 sm.process_event(event3()); 时,状态机会从 state3 转换回 state1。
这个例子比较简单,但是可以帮助你了解如何使用 boost 库来实现一个简单的状态机。
相关推荐
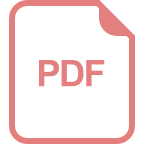
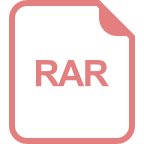
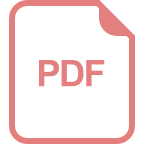














