读取文件nameList.txt中的候选人名单,随机抽取三位数候选人作为获奖者,将获奖人信息输出到屏幕同时保存到文件winner.txt
时间: 2024-03-10 15:49:45 浏览: 21
好的,我可以编写一个Python程序来完成这个任务。请确保nameList.txt文件与程序在同一个目录下,并且文件中每行只包含一个候选人的姓名。
以下是程序的代码:
```python
import random
# 读取候选人名单
with open("nameList.txt", "r") as f:
candidates = f.readlines()
# 随机抽取三位获奖者
winners = random.sample(candidates, 3)
# 输出获奖者信息到屏幕并保存到文件
with open("winner.txt", "w") as f:
for winner in winners:
f.write(winner)
print("恭喜{}获奖!".format(winner.strip()))
```
运行程序后,获奖者信息会被输出到屏幕并保存到winner.txt文件中。请注意,程序中使用了Python的随机抽样函数`random.sample()`来从候选人列表中随机抽取三位获奖者。
相关问题
用c++编写:读取文件nameList中候选人名单,随机抽取三位候选人作为获奖者,将获奖人信息输出到屏幕上同时保存到文件winner.txt
以下是用c++编写的代码:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
int main()
{
// 打开名单文件
ifstream ifs("nameList.txt");
if (!ifs.is_open()) {
cerr << "Failed to open nameList.txt" << endl;
return 1;
}
// 读取名单并存储到vector中
vector<string> names;
string name;
while (getline(ifs, name)) {
names.push_back(name);
}
// 关闭文件
ifs.close();
// 随机打乱vector中的元素
random_shuffle(names.begin(), names.end());
// 选择前三个元素作为获奖者
vector<string> winners(names.begin(), names.begin() + 3);
// 输出获奖者信息到屏幕上
cout << "Winners:\n";
for (const auto& winner : winners) {
cout << winner << endl;
}
// 将获奖者信息保存到文件winner.txt中
ofstream ofs("winner.txt");
if (!ofs.is_open()) {
cerr << "Failed to create winner.txt" << endl;
return 1;
}
for (const auto& winner : winners) {
ofs << winner << endl;
}
// 关闭文件
ofs.close();
return 0;
}
```
在上述代码中,我们首先打开名单文件,读取其中的候选人名单,并存储到vector中。然后使用C++中的random_shuffle函数打乱vector中的元素,接着选择前三个元素作为获奖者。将获奖者信息输出到屏幕上,并且将获奖者信息保存到文件winner.txt中。最后关闭文件并退出程序。
namelist.wps中fdda怎么设置
`namelist.wps`文件中的`fdda`参数是用来控制有限区域外的气象场对有限区域内气象场的影响的。`fdda`参数的设置涉及到以下几个参数:
- `fdda`:控制是否启用 FDDA(分析增量)功能。设置为`1`表示启用,设置为`0`表示禁用。
- `gfdda_interval_m`:FDDA 分析的时间间隔,单位为分钟。
- `gfdda_end_h`:FDDA 分析结束的模拟时间,单位为小时。
- `gfdda_inname`:FDDA 输入文件的路径和文件名。
- `gfdda_outname`:FDDA 输出文件的路径和文件名。
- `gfdda_nx`、`gfdda_ny`、`gfdda_nz`:FDDA 输入数据的水平和垂直分辨率。
- `gfdda_lat1`、`gfdda_lat2`、`gfdda_lon1`、`gfdda_lon2`:FDDA 输入数据的经纬度范围。
- `gfdda_interval_m`、`gfdda_parent_grid_ratio`:FDDA 网格比率,用于将 FDDA 数据映射到模拟网格。
在使用`fdda`参数时,需要注意以下几点:
- FDDA 输入文件必须包含与模拟网格相同的水平和垂直分辨率。
- FDDA 输入文件必须涵盖模拟区域外部的区域。
- FDDA 输入文件必须包含与模拟起始时间相对应的分析时间步长。
- FDDA 输入文件必须包含与模拟起始时间和模拟结束时间之间的所有时间步长。
相关推荐
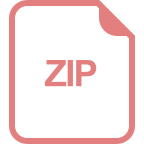













