解释代码意思img = img*mask2[:, :, np.newaxis]
时间: 2024-05-27 22:11:27 浏览: 17
这行代码的作用是将二维的掩码数组(mask2)转换为三维数组,并将其与三维的图像数组(img)进行逐元素相乘操作。
具体来说,mask2[:, :, np.newaxis]的结果是将二维的掩码数组(mask2)在第三个维度上扩展为一个长度为1的新维度,因此其形状变为(m, n, 1)。
然后,img和mask2[:, :, np.newaxis]进行逐元素相乘,即将img的每个元素与mask2的对应元素相乘,得到的结果是一个形状与img相同的三维数组。这个数组的第三个维度与mask2的新维度一样,都是1,因此相当于将mask2的值应用到了img的每个通道上,从而实现了掩码操作。
相关问题
解释下列代码意思img = img*mask2[:, :, np.newaxis]
这行代码将一个三维的图像数组(img)与一个二维的掩膜数组(mask2)相乘,并在掩膜数组上增加一个新的维度。
这个新的维度使掩膜数组与图像数组的维数相同,以便可以进行元素级别的乘法运算。
具体来说,乘法运算会将掩膜数组中为1的位置对应的图像数组中的像素值保留下来,而将掩膜数组中为0的位置对应的图像数组中的像素值置为0。
因此,这行代码的作用是将图像数组中不需要的区域(在掩膜数组中为0的区域)置为0,以此来实现图像的掩膜处理。
利用超像素优化deblurgan-v2的pytorch代码
DeblurGAN-v2 是一种图像去模糊的深度学习模型,可用于将模糊图像转换为清晰图像。在该模型中,使用了超像素技术来提高去模糊的效果。下面是利用超像素优化DeblurGAN-v2的PyTorch代码:
首先,需要安装以下依赖库:
```
pip install opencv-python
pip install scikit-image
pip install numpy
pip install torch
pip install torchvision
pip install pydensecrf
```
然后,加载DeblurGAN-v2模型和测试图像,并生成超像素:
```python
import cv2
import torch
import numpy as np
from skimage.segmentation import slic
from skimage.segmentation import mark_boundaries
from skimage.color import rgb2gray
from models.networks import define_G
from options.test_options import TestOptions
from util import util
from pydensecrf.densecrf import DenseCRF2D
# 加载模型
opt = TestOptions().parse()
opt.nThreads = 1
opt.batchSize = 1
opt.serial_batches = True
opt.no_flip = True
model = define_G(opt)
util.load_checkpoint(model, opt.pretrained)
# 加载测试图像
img_path = 'path/to/test/image'
img = cv2.imread(img_path)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
h, w, c = img.shape
# 生成超像素
segments = slic(img, n_segments=100, sigma=5, compactness=10)
```
接下来,将每个超像素作为输入,运行DeblurGAN-v2模型进行去模糊:
```python
# 对每个超像素进行去模糊
result = np.zeros((h, w, c), dtype=np.float32)
for i in np.unique(segments):
mask = (segments == i).astype(np.uint8)
masked_img = cv2.bitwise_and(img, img, mask=mask)
if np.sum(mask) > 0:
masked_img = masked_img[np.newaxis, :, :, :]
masked_img = torch.from_numpy(masked_img.transpose((0, 3, 1, 2))).float()
with torch.no_grad():
output = model(masked_img)
output = output.cpu().numpy()
output = output.transpose((0, 2, 3, 1))
output = np.squeeze(output)
result += output * mask[:, :, np.newaxis]
# 对结果进行后处理
result /= 255.0
result = np.clip(result, 0, 1)
result = (result * 255).astype(np.uint8)
```
最后,使用密集条件随机场(DenseCRF)算法对结果进行后处理,以进一步提高去模糊的效果:
```python
# 使用DenseCRF算法进行后处理
d = DenseCRF2D(w, h, 2)
result_softmax = np.stack([result, 255 - result], axis=0)
result_softmax = result_softmax.astype(np.float32) / 255.0
unary = -np.log(result_softmax)
unary = unary.reshape((2, -1))
d.setUnaryEnergy(unary)
d.addPairwiseGaussian(sxy=5, compat=3)
d.addPairwiseBilateral(sxy=20, srgb=3, rgbim=img, compat=10)
q = d.inference(5)
q = np.argmax(np.array(q), axis=0).reshape((h, w))
result = q * 255
```
完整代码如下:
```python
import cv2
import torch
import numpy as np
from skimage.segmentation import slic
from skimage.segmentation import mark_boundaries
from skimage.color import rgb2gray
from models.networks import define_G
from options.test_options import TestOptions
from util import util
from pydensecrf.densecrf import DenseCRF2D
# 加载模型
opt = TestOptions().parse()
opt.nThreads = 1
opt.batchSize = 1
opt.serial_batches = True
opt.no_flip = True
model = define_G(opt)
util.load_checkpoint(model, opt.pretrained)
# 加载测试图像
img_path = 'path/to/test/image'
img = cv2.imread(img_path)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
h, w, c = img.shape
# 生成超像素
segments = slic(img, n_segments=100, sigma=5, compactness=10)
# 对每个超像素进行去模糊
result = np.zeros((h, w, c), dtype=np.float32)
for i in np.unique(segments):
mask = (segments == i).astype(np.uint8)
masked_img = cv2.bitwise_and(img, img, mask=mask)
if np.sum(mask) > 0:
masked_img = masked_img[np.newaxis, :, :, :]
masked_img = torch.from_numpy(masked_img.transpose((0, 3, 1, 2))).float()
with torch.no_grad():
output = model(masked_img)
output = output.cpu().numpy()
output = output.transpose((0, 2, 3, 1))
output = np.squeeze(output)
result += output * mask[:, :, np.newaxis]
# 对结果进行后处理
result /= 255.0
result = np.clip(result, 0, 1)
result = (result * 255).astype(np.uint8)
# 使用DenseCRF算法进行后处理
d = DenseCRF2D(w, h, 2)
result_softmax = np.stack([result, 255 - result], axis=0)
result_softmax = result_softmax.astype(np.float32) / 255.0
unary = -np.log(result_softmax)
unary = unary.reshape((2, -1))
d.setUnaryEnergy(unary)
d.addPairwiseGaussian(sxy=5, compat=3)
d.addPairwiseBilateral(sxy=20, srgb=3, rgbim=img, compat=10)
q = d.inference(5)
q = np.argmax(np.array(q), axis=0).reshape((h, w))
result = q * 255
# 显示结果
result = cv2.cvtColor(result, cv2.COLOR_RGB2BGR)
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
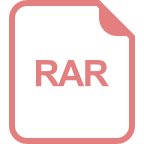
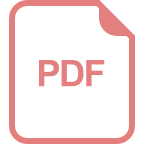












