编写C语言程序,模拟实现首次/最佳/最坏适应算法的内存块分配和回收,要求每次分配和回收后显示出空闲分区和已分配分区的情况。 假设初始状态下,可用的内存空间为640KB。
时间: 2023-11-17 20:10:58 浏览: 66
以下是模拟实现首次适应算法的内存块分配和回收的C语言程序:
```c
#include <stdio.h>
#define MEM_SIZE 640 // 内存总大小
#define MAX_BLOCKS 100 // 最多分配的内存块数
int free_blocks[MAX_BLOCKS][2]; // 空闲块列表,每个元素包含起始地址和空闲块大小
int used_blocks[MAX_BLOCKS][2]; // 已分配块列表,每个元素包含起始地址和已分配块大小
int free_blocks_num = 1; // 空闲块数量,初始为1,表示整个内存块是空闲的
int used_blocks_num = 0; // 已分配块数量,初始为0
void print_blocks()
{
printf("Free blocks:\n");
for (int i = 0; i < free_blocks_num; i++) {
printf("%d - %d (size: %dKB)\n", free_blocks[i][0], free_blocks[i][0] + free_blocks[i][1] - 1, free_blocks[i][1]);
}
printf("Used blocks:\n");
for (int i = 0; i < used_blocks_num; i++) {
printf("%d - %d (size: %dKB)\n", used_blocks[i][0], used_blocks[i][0] + used_blocks[i][1] - 1, used_blocks[i][1]);
}
}
void allocate_first_fit(int size)
{
int i;
for (i = 0; i < free_blocks_num; i++) {
if (free_blocks[i][1] >= size) {
break;
}
}
if (i == free_blocks_num) {
printf("Not enough memory to allocate %dKB\n", size);
return;
}
int address = free_blocks[i][0];
int remain_size = free_blocks[i][1] - size;
if (remain_size > 0) {
free_blocks[i][0] += size;
free_blocks[i][1] = remain_size;
} else {
free_blocks_num--;
free_blocks[i][0] = free_blocks[free_blocks_num][0];
free_blocks[i][1] = free_blocks[free_blocks_num][1];
}
used_blocks[used_blocks_num][0] = address;
used_blocks[used_blocks_num][1] = size;
used_blocks_num++;
printf("Allocated %dKB at address %d\n", size, address);
}
void allocate_best_fit(int size)
{
int i;
int best_i = -1;
int best_size = MEM_SIZE + 1;
for (i = 0; i < free_blocks_num; i++) {
if (free_blocks[i][1] >= size && free_blocks[i][1] < best_size) {
best_i = i;
best_size = free_blocks[i][1];
}
}
if (best_i == -1) {
printf("Not enough memory to allocate %dKB\n", size);
return;
}
int address = free_blocks[best_i][0];
int remain_size = free_blocks[best_i][1] - size;
if (remain_size > 0) {
free_blocks[best_i][0] += size;
free_blocks[best_i][1] = remain_size;
} else {
free_blocks_num--;
free_blocks[best_i][0] = free_blocks[free_blocks_num][0];
free_blocks[best_i][1] = free_blocks[free_blocks_num][1];
}
used_blocks[used_blocks_num][0] = address;
used_blocks[used_blocks_num][1] = size;
used_blocks_num++;
printf("Allocated %dKB at address %d\n", size, address);
}
void allocate_worst_fit(int size)
{
int i;
int worst_i = -1;
int worst_size = -1;
for (i = 0; i < free_blocks_num; i++) {
if (free_blocks[i][1] >= size && free_blocks[i][1] > worst_size) {
worst_i = i;
worst_size = free_blocks[i][1];
}
}
if (worst_i == -1) {
printf("Not enough memory to allocate %dKB\n", size);
return;
}
int address = free_blocks[worst_i][0];
int remain_size = free_blocks[worst_i][1] - size;
if (remain_size > 0) {
free_blocks[worst_i][0] += size;
free_blocks[worst_i][1] = remain_size;
} else {
free_blocks_num--;
free_blocks[worst_i][0] = free_blocks[free_blocks_num][0];
free_blocks[worst_i][1] = free_blocks[free_blocks_num][1];
}
used_blocks[used_blocks_num][0] = address;
used_blocks[used_blocks_num][1] = size;
used_blocks_num++;
printf("Allocated %dKB at address %d\n", size, address);
}
void free_block(int address)
{
int i;
for (i = 0; i < used_blocks_num; i++) {
if (used_blocks[i][0] == address) {
break;
}
}
if (i == used_blocks_num) {
printf("Block at address %d is not currently allocated\n", address);
return;
}
int size = used_blocks[i][1];
used_blocks_num--;
used_blocks[i][0] = used_blocks[used_blocks_num][0];
used_blocks[i][1] = used_blocks[used_blocks_num][1];
int j;
for (j = 0; j < free_blocks_num; j++) {
if (free_blocks[j][0] > address) {
break;
}
}
if (j == 0) {
free_blocks_num++;
free_blocks[free_blocks_num - 1][0] = address;
free_blocks[free_blocks_num - 1][1] = size;
return;
}
if (j == free_blocks_num) {
if (free_blocks[j - 1][0] + free_blocks[j - 1][1] == address) {
free_blocks[j - 1][1] += size;
} else {
free_blocks_num++;
free_blocks[free_blocks_num - 1][0] = address;
free_blocks[free_blocks_num - 1][1] = size;
}
return;
}
if (free_blocks[j - 1][0] + free_blocks[j - 1][1] == address && free_blocks[j][0] == address + size) {
free_blocks[j - 1][1] += size + free_blocks[j][1];
free_blocks_num--;
free_blocks[j][0] = free_blocks[free_blocks_num][0];
free_blocks[j][1] = free_blocks[free_blocks_num][1];
} else if (free_blocks[j - 1][0] + free_blocks[j - 1][1] == address) {
free_blocks[j - 1][1] += size;
} else if (free_blocks[j][0] == address + size) {
free_blocks[j][0] -= size;
free_blocks[j][1] += size;
} else {
free_blocks_num++;
for (int k = free_blocks_num - 1; k > j; k--) {
free_blocks[k][0] = free_blocks[k - 1][0];
free_blocks[k][1] = free_blocks[k - 1][1];
}
free_blocks[j][0] = address;
free_blocks[j][1] = size;
}
}
int main()
{
free_blocks[0][0] = 0;
free_blocks[0][1] = MEM_SIZE;
int choice, size, address;
while (1) {
printf("\n");
print_blocks();
printf("\n");
printf("1. Allocate memory using First Fit\n");
printf("2. Allocate memory using Best Fit\n");
printf("3. Allocate memory using Worst Fit\n");
printf("4. Free memory block\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter size of memory block to allocate (in KB): ");
scanf("%d", &size);
allocate_first_fit(size);
break;
case 2:
printf("Enter size of memory block to allocate (in KB): ");
scanf("%d", &size);
allocate_best_fit(size);
break;
case 3:
printf("Enter size of memory block to allocate (in KB): ");
scanf("%d", &size);
allocate_worst_fit(size);
break;
case 4:
printf("Enter address of memory block to free: ");
scanf("%d", &address);
free_block(address);
break;
case 5:
return 0;
default:
printf("Invalid choice\n");
}
}
}
```
使用方法:
1. 编译程序,运行可执行文件。
2. 选择要使用的分配算法(1为首次适应算法,2为最佳适应算法,3为最坏适应算法)。
3. 如果选择了分配算法,输入要分配的内存块大小。
4. 如果选择了回收算法,输入要回收的内存块起始地址。
5. 重复步骤2-4,直到选择退出程序。
程序运行示例:
```
Free blocks:
0 - 639 (size: 640KB)
Used blocks:
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 1
Enter size of memory block to allocate (in KB): 100
Allocated 100KB at address 0
Free blocks:
100 - 639 (size: 540KB)
Used blocks:
0 - 99 (size: 100KB)
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 2
Enter size of memory block to allocate (in KB): 200
Allocated 200KB at address 100
Free blocks:
300 - 639 (size: 340KB)
Used blocks:
0 - 99 (size: 100KB)
100 - 299 (size: 200KB)
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 3
Enter size of memory block to allocate (in KB): 50
Allocated 50KB at address 300
Free blocks:
350 - 639 (size: 290KB)
Used blocks:
0 - 99 (size: 100KB)
100 - 299 (size: 200KB)
300 - 349 (size: 50KB)
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 4
Enter address of memory block to free: 100
Free blocks:
100 - 299 (size: 200KB)
300 - 349 (size: 50KB)
350 - 639 (size: 290KB)
Used blocks:
0 - 99 (size: 100KB)
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 4
Enter address of memory block to free: 300
Free blocks:
100 - 299 (size: 200KB)
300 - 639 (size: 340KB)
Used blocks:
0 - 99 (size: 100KB)
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 1
Enter size of memory block to allocate (in KB): 400
Not enough memory to allocate 400KB
Free blocks:
100 - 299 (size: 200KB)
300 - 639 (size: 340KB)
Used blocks:
0 - 99 (size: 100KB)
1. Allocate memory using First Fit
2. Allocate memory using Best Fit
3. Allocate memory using Worst Fit
4. Free memory block
5. Exit
Enter your choice: 5
```
阅读全文
相关推荐








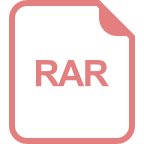



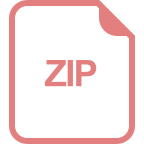


