stream list<object> 遍历 List<String>去重
时间: 2023-09-26 13:09:25 浏览: 43
可以使用LINQ库中的Distinct()方法来去重,示例代码如下:
```csharp
List<object> stream = new List<object>();
List<string> stringList = new List<string>();
// 假设stream已经被初始化并填充
// 假设stringList已经被初始化并填充
var distinctStringList = stringList.Distinct();
foreach (object obj in stream)
{
foreach (string str in distinctStringList)
{
// 在这里处理obj和str
}
}
```
在上面的代码中,我们首先使用Distinct()方法来去重stringList中的元素,并将结果存储在distinctStringList变量中。然后,我们使用两个嵌套的foreach循环来遍历stream和distinctStringList,并在内部处理它们。
相关问题
List<Object> 转 List<String> java
可以使用Java 8的Stream API将List<Object>转换为List<String>。以下是一个示例代码:
```java
List<Object> objectList = Arrays.asList("Hello", 123, true, "World", 456);
List<String> stringList = objectList.stream()
.map(Object::toString)
.collect(Collectors.toList());
```
在这个例子中,`objectList`包含了不同类型的对象,我们将它们转换为字符串类型。我们首先使用`stream()`方法将List转换为Stream,然后使用`map()`方法将每个对象转换为字符串。最后,我们使用`collect()`方法将结果收集到一个新的List中。
如果你使用的是Java 7或更早的版本,你可以使用传统的for循环遍历List并将每个Object转换为String,然后添加到新的List中。以下是一个示例代码:
```java
List<Object> objectList = Arrays.asList("Hello", 123, true, "World", 456);
List<String> stringList = new ArrayList<String>();
for (Object obj : objectList) {
stringList.add(obj.toString());
}
```
在这个例子中,我们首先创建一个空的List<String>,然后使用for循环遍历List<Object>,将每个对象转换为字符串并添加到新的List中。
List<String>怎么强转为List<Object>
在Java中,不可以直接将List<String>强制转换为List<Object>。这是因为泛型在Java中是不可逆的,即使String是Object的子类,List<String>也不是List<Object>的子类。所以在进行强制类型转换时会出现编译错误。
解决方法有两种:
1. 使用遍历将List<String>转换为List<Object>。可以使用Java 8的Stream API来实现,首先将List<String>转为Stream,然后通过map操作将每个元素转为Object,最后使用collect操作将Stream转为List<Object>。
```
List<String> sun = Lists.newArrayList("a");
List<Object> father = sun.stream()
.map(e -> (Object)e)
.collect(Collectors.toList());
```
2. 创建一个新的List<Object>,然后将List<String>中的元素逐个添加到新的List<Object>中。这种方法需要手动遍历List<String>,并将每个元素转为Object,然后添加到新的List<Object>中。
```
List<String> sun = Lists.newArrayList("a");
List<Object> father = new ArrayList<>();
for(String s : sun) {
father.add((Object)s);
}
```
相关推荐
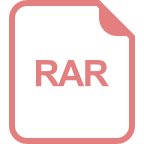
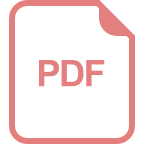
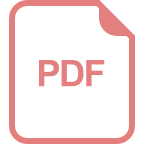












