def win_car(car, contestant, monty, strategy): # Parameters # car, which is either 1, 2 or 3, represents the door behind which is a car # contestant, which is either 1, 2 or 3, represents the door that contestant chooses # monty, which is either 1, 2 or 3, represents the door that the host opens # strategy, a string which is either 'switch' or 'noswitch' # Returns # a boolean value. True for winning a car and False for winning a goat # write your code below this line。请帮我完成需要填写的代码
时间: 2024-03-27 11:39:36 浏览: 14
```python
def win_car(car, contestant, monty, strategy):
if strategy == 'noswitch':
return contestant == car
elif strategy == 'switch':
doors = [1, 2, 3]
doors.remove(monty)
doors.remove(contestant)
contestant = doors[0]
return contestant == car
else:
print('Invalid strategy!')
return None
```
这段代码首先判断 `strategy` 的值是 `'noswitch'` 还是 `'switch'`。如果是 `'noswitch'`,那么只需要判断选手最开始选择的门是否是 `car` 即可。如果是 `'switch'`,则需要先将 `monty` 打开的门从列表中删除,然后再将选手最开始选择的门也从列表中删除。此时,列表中只剩下了一扇门,即选手可以选择的另一扇门。选手可以选择这扇门,然后判断是否获得了汽车。最后,如果 `strategy` 的值既不是 `'noswitch'` 也不是 `'switch'`,那么将输出一条错误消息,并返回 `None`。
相关问题
def monty_choice(car, contestant): # this method simulates Monty opening an door behind which there is a goat # Parameters # car, which is either 1, 2 or 3, represents the door behind which is a car # contestant, which is either 1, 2 or 3, represents the door that contestant chooses # Returns # an integer, which is 1,2 or 3, represents the door that host chooses to open # write your code below this line
Here's one possible implementation of the `monty_choice` function in Python:
```python
def monty_choice(car, contestant):
# find the door that Monty can open
options = [1, 2, 3]
options.remove(car)
if contestant in options:
options.remove(contestant)
door_to_open = random.choice(options)
return door_to_open
```
This function takes in two parameters: `car` and `contestant`, which represent the door behind which is a car and the door that the contestant chooses, respectively. It returns an integer, which represents the door that Monty chooses to open.
The function first creates a list of all possible doors (`options`) and removes the door containing the car. If the contestant has not already chosen the car door, it also removes the contestant's chosen door. The function then randomly selects one of the remaining doors to open (`door_to_open`) and returns it.
counter = 0 for i in range(n): car = random_door() contestant = random_door() monty = monty_choice(car, contestant) if win_car(car, contestant, monty, strategy): counter += 1 return counter, n - counter这段代码什么意思,请详细解释一下
这段代码是一个模拟 Monty Hall 问题的函数,该问题是一个著名的概率问题。问题的描述是这样的:有三扇门,其中一扇门后面有一辆汽车,另外两扇门后面是山羊。参赛者先选择一扇门,然后 Monty 会打开一扇有山羊的门,问参赛者是否要更换自己的选择,以增加获得汽车的概率。
现在来解释代码的具体意思:
- 首先,代码中定义了一个计数器 counter,用于记录参赛者获得汽车的次数。
- 然后,代码使用 for 循环,重复进行 n 次模拟。
- 在每次模拟中,代码随机选择一扇门作为汽车的位置(变量名为 car),随机选择一扇门作为参赛者的选择(变量名为 contestant),然后根据 Monty 的策略选择一扇门打开(变量名为 monty)。
- 最后,根据参赛者的策略(变量名为 strategy),判断参赛者是否获得了汽车,如果获得了,让计数器 counter 加 1。
- 最终,函数返回两个值,分别是参赛者获得汽车的次数和未获得汽车的次数。
相关推荐
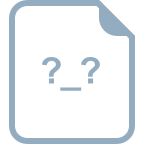
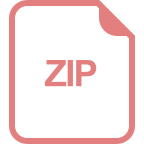











