基于eclipse mapreduce hadoop的天气统计代码
时间: 2023-07-11 22:33:44 浏览: 69
以下是基于Eclipse和Hadoop实现的天气统计代码示例:
1. 首先,创建一个Java项目并在项目中创建一个MapReduce类。
```java
package com.example.weather;
import java.io.IOException;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class WeatherCount {
public static class WeatherMapper extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
public void map(Object key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
String weather = fields[2];
context.write(new Text(weather), one);
}
}
public static class WeatherReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
public static void main(String[] args) throws Exception {
Job job = new Job();
job.setJarByClass(WeatherCount.class);
job.setMapperClass(WeatherMapper.class);
job.setCombinerClass(WeatherReducer.class);
job.setReducerClass(WeatherReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
2. 在该代码中,我们定义了一个Mapper类和一个Reducer类,其中Mapper类用于从输入数据中提取天气信息,而Reducer类用于将具有相同天气的数据进行聚合。
3. 在main函数中,我们设置了Mapper和Reducer类,指定了输入和输出路径,并使用job.waitForCompletion()方法来启动MapReduce作业。
4. 编译并打包该代码,然后在Hadoop集群上运行该作业。假设输入数据文件为input.txt,可以使用以下命令来运行作业:
```bash
$ hadoop jar WeatherCount.jar com.example.weather.WeatherCount input.txt output
```
5. 运行完成后,输出结果将存储在output文件夹中。可以使用以下命令查看输出结果:
```bash
$ hdfs dfs -cat output/*
```
以上代码示例可以自行修改和扩展,以适应不同的数据集和需求。
相关推荐
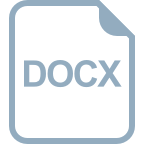
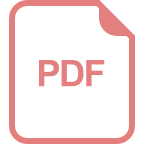
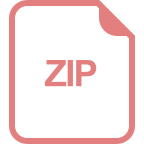
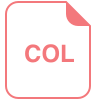
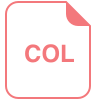












