结合上课讲授内容,从https://ssr1.scrape.center网站上分别爬取10个 页面的网页源代码,使用正则表达式提取共100部电影的电影名称, 类型,制作产地,播放时长,发布日期及评分的数据,保存到.csv文 件中
时间: 2024-06-09 09:08:46 浏览: 160
好的,我可以帮你完成这个任务。我们需要使用 Python 的 requests 库来爬取网页源代码,使用正则表达式来提取数据,并使用 pandas 库将数据保存到 CSV 文件中。
首先,我们需要安装 requests 和 pandas 库。你可以使用以下命令来安装它们:
```
pip install requests pandas
```
接下来,我们可以开始编写 Python 代码。以下是完整的代码:
```python
import re
import requests
import pandas as pd
# 定义正则表达式
name_pattern = re.compile(r'<h2>\s*<a[^>]*>(.*?)</a>', re.S)
info_pattern = re.compile(r'<div class="channel-detail movie-item-title" title="(.*?)">', re.S)
score_pattern = re.compile(r'<div class="channel-detail channel-detail-orange"><i class="integer">(.*?)</i><i class="fraction">(.*?)</i></div>', re.S)
# 定义函数来获取网页源代码并提取数据
def get_movie_data(url):
# 发送 GET 请求并获取网页源代码
response = requests.get(url)
html = response.text
# 使用正则表达式提取电影名称、类型、制作产地、播放时长、发布日期和评分
names = name_pattern.findall(html)
infos = info_pattern.findall(html)
scores = score_pattern.findall(html)
data = []
for i in range(len(names)):
name = names[i].strip()
info = infos[i].strip().split('/')
score = float(scores[i][0] + '.' + scores[i][1])
movie_data = {
'名称': name,
'类型': info[0],
'制作产地': info[1],
'播放时长': info[2],
'发布日期': info[3],
'评分': score
}
data.append(movie_data)
return data
# 定义要爬取的网页 URL
urls = [
'https://ssr1.scrape.center/page/1',
'https://ssr1.scrape.center/page/2',
'https://ssr1.scrape.center/page/3',
'https://ssr1.scrape.center/page/4',
'https://ssr1.scrape.center/page/5',
'https://ssr1.scrape.center/page/6',
'https://ssr1.scrape.center/page/7',
'https://ssr1.scrape.center/page/8',
'https://ssr1.scrape.center/page/9',
'https://ssr1.scrape.center/page/10'
]
# 爬取数据并保存到 CSV 文件
data = []
for url in urls:
data += get_movie_data(url)
df = pd.DataFrame(data)
df.to_csv('movies.csv', index=False, encoding='utf-8-sig')
```
代码中定义了三个正则表达式,分别用于提取电影名称、类型、制作产地、播放时长、发布日期和评分。然后,我们定义了一个 `get_movie_data` 函数来获取网页源代码并提取数据。该函数接受一个 URL 参数,并返回一个包含电影数据的列表。
接下来,我们定义了要爬取的网页 URL 列表,并循环遍历每个 URL,调用 `get_movie_data` 函数来获取数据,并将所有数据保存到一个列表中。最后,我们使用 pandas 库将数据保存到 CSV 文件中。
请注意,我们使用了 `utf-8-sig` 编码来保存 CSV 文件,因为该编码可以确保在 Excel 中正确显示中文字符。
希望这可以帮助你完成任务!
阅读全文
相关推荐
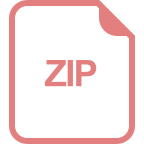

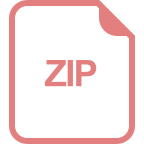
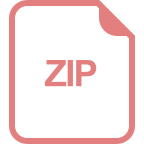
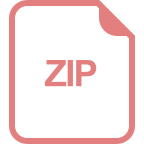


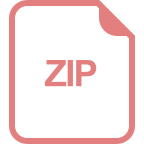
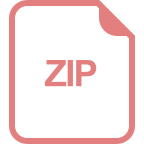
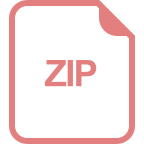
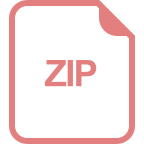
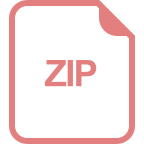
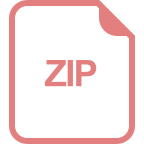
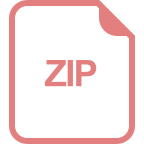
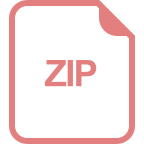
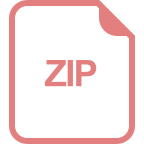
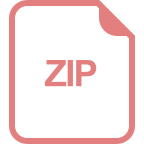