用winform写一个坦克大战
时间: 2024-06-09 16:05:12 浏览: 45
这里是一个简单的WinForms坦克大战游戏的实现方法:
1. 创建一个新的Windows Forms应用程序项目
2. 在窗体上添加一个PictureBox控件,用于显示游戏场景
3. 创建一个Tank类,包括坦克的位置、方向、速度、生命值等信息,并实现移动、开炮等方法
4. 创建一个Bullet类,包括子弹的位置、方向、速度等信息,并实现移动等方法
5. 在窗体中添加一个Timer控件,用于定期刷新游戏场景
6. 在Timer的Tick事件中,更新所有坦克和子弹的位置,并检测是否有坦克被击中
7. 在窗体的KeyPress事件中,判断用户按下的键盘按钮,并控制玩家坦克移动或开炮
8. 在窗体的Paint事件中,绘制所有坦克和子弹的图像
下面是大致的代码实现:
Tank类:
```csharp
public class Tank
{
public Point Location { get; set; }
public int Direction { get; set; }
public int Speed { get; set; }
public int Health { get; set; }
public Image Image { get; set; }
public Tank(Point location, Image image)
{
Location = location;
Direction = 0;
Speed = 5;
Health = 100;
Image = image;
}
public void Move()
{
int x = Location.X;
int y = Location.Y;
switch (Direction)
{
case 0: y -= Speed; break;
case 1: x += Speed; break;
case 2: y += Speed; break;
case 3: x -= Speed; break;
}
Location = new Point(x, y);
}
public Bullet Fire()
{
Point location = new Point(Location.X + Image.Width / 2, Location.Y + Image.Height / 2);
return new Bullet(location, Direction);
}
}
```
Bullet类:
```csharp
public class Bullet
{
public Point Location { get; set; }
public int Direction { get; set; }
public int Speed { get; set; }
public Image Image { get; set; }
public Bullet(Point location, int direction)
{
Location = location;
Direction = direction;
Speed = 10;
Image = Properties.Resources.bullet;
}
public void Move()
{
int x = Location.X;
int y = Location.Y;
switch (Direction)
{
case 0: y -= Speed; break;
case 1: x += Speed; break;
case 2: y += Speed; break;
case 3: x -= Speed; break;
}
Location = new Point(x, y);
}
}
```
窗体的代码:
```csharp
public partial class Form1 : Form
{
private Tank playerTank;
private List<Tank> enemyTanks;
private List<Bullet> bullets;
private Random random;
public Form1()
{
InitializeComponent();
playerTank = new Tank(new Point(100, 100), Properties.Resources.player);
enemyTanks = new List<Tank>();
bullets = new List<Bullet>();
random = new Random();
for (int i = 0; i < 3; i++)
{
enemyTanks.Add(new Tank(new Point(random.Next(500), random.Next(500)), Properties.Resources.enemy));
}
timer1.Start();
}
private void Form1_KeyPress(object sender, KeyPressEventArgs e)
{
switch (e.KeyChar)
{
case 'w': playerTank.Direction = 0; break;
case 'd': playerTank.Direction = 1; break;
case 's': playerTank.Direction = 2; break;
case 'a': playerTank.Direction = 3; break;
case ' ': bullets.Add(playerTank.Fire()); break;
}
}
private void timer1_Tick(object sender, EventArgs e)
{
playerTank.Move();
foreach (Tank enemy in enemyTanks)
{
enemy.Direction = random.Next(4);
enemy.Move();
if (random.Next(10) == 0)
{
bullets.Add(enemy.Fire());
}
}
foreach (Bullet bullet in bullets)
{
bullet.Move();
}
// 检测是否有坦克被击中
for (int i = bullets.Count - 1; i >= 0; i--)
{
Bullet bullet = bullets[i];
if (bullet.Location.X < 0 || bullet.Location.X > pictureBox1.Width || bullet.Location.Y < 0 || bullet.Location.Y > pictureBox1.Height)
{
bullets.RemoveAt(i);
continue;
}
if (bullet.Direction == 0 && bullet.Location.Y < playerTank.Location.Y + playerTank.Image.Height / 2 && bullet.Location.Y > playerTank.Location.Y && bullet.Location.X > playerTank.Location.X && bullet.Location.X < playerTank.Location.X + playerTank.Image.Width)
{
playerTank.Health -= 10;
bullets.RemoveAt(i);
}
foreach (Tank enemy in enemyTanks)
{
if (bullet.Direction == 2 && bullet.Location.Y > enemy.Location.Y + enemy.Image.Height / 2 && bullet.Location.Y < enemy.Location.Y + enemy.Image.Height && bullet.Location.X > enemy.Location.X && bullet.Location.X < enemy.Location.X + enemy.Image.Width)
{
enemy.Health -= 10;
bullets.RemoveAt(i);
}
}
}
// 移除生命值为0的坦克
enemyTanks.RemoveAll(t => t.Health <= 0);
// 绘制游戏场景
pictureBox1.Invalidate();
}
private void pictureBox1_Paint(object sender, PaintEventArgs e)
{
e.Graphics.DrawImage(playerTank.Image, playerTank.Location);
foreach (Tank enemy in enemyTanks)
{
e.Graphics.DrawImage(enemy.Image, enemy.Location);
}
foreach (Bullet bullet in bullets)
{
e.Graphics.DrawImage(bullet.Image, bullet.Location);
}
e.Graphics.DrawString("Health: " + playerTank.Health, Font, Brushes.Red, 0, 0);
}
}
```
这只是一个简单的实现,你可以根据自己的需求和兴趣对其进行更多的扩展和改进。
阅读全文
相关推荐
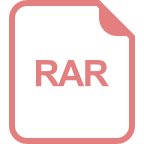


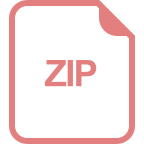
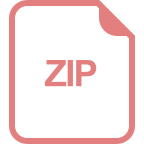
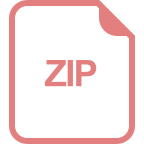
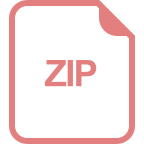
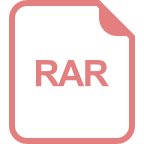
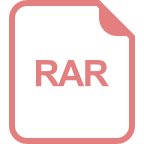
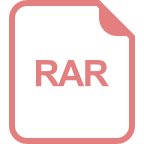
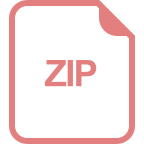
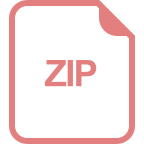
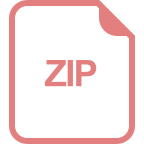
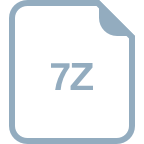
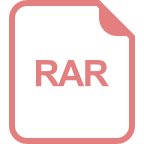
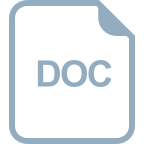