使用Vue开发一个简单的todoList
时间: 2024-05-05 22:18:32 浏览: 9
Sure, I can help you with that! To develop a simple to-do list using Vue, we will need to first create a new Vue instance and then set up the data and methods for the app. Here's an example:
```
<!DOCTYPE html>
<html>
<head>
<title>Vue Todo List</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h1>Todo List</h1>
<input v-model="newTodo" type="text" placeholder="Add new task">
<button v-on:click="addTodo">Add</button>
<ul>
<li v-for="(todo, index) in todos" :key="index">
{{ todo }}
<button v-on:click="deleteTodo(index)">Delete</button>
</li>
</ul>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
newTodo: '',
todos: []
},
methods: {
addTodo: function() {
if (this.newTodo !== '') {
this.todos.push(this.newTodo);
this.newTodo = '';
}
},
deleteTodo: function(index) {
this.todos.splice(index, 1);
}
}
})
</script>
</body>
</html>
```
In this code, we use the `v-model` directive to bind the input field to the `newTodo` data property, and the `v-for` directive to loop through the `todos` array and display each item in the list. We also create methods to add and delete items from the list. Let me know if you have any questions!
相关推荐
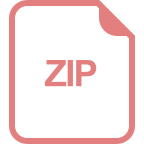
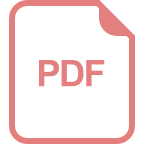











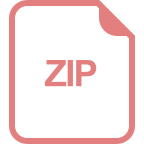
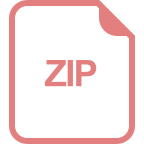
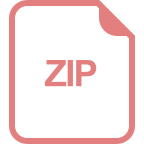