用c++ opencv实现mat的exp运算
时间: 2024-03-09 15:47:05 浏览: 20
在C++中,我们可以使用OpenCV库中的cv::exp函数来实现Mat的exp运算。该函数的原型如下:
```cpp
void cv::exp(InputArray src, OutputArray dst);
```
其中,src是输入的Mat对象,dst是输出的Mat对象。
下面是一个示例代码,实现对Mat对象的exp运算:
```cpp
#include <opencv2/core.hpp>
#include <opencv2/highgui.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main()
{
Mat input = imread("test.jpg", IMREAD_GRAYSCALE);
Mat output;
cv::exp(input, output);
imshow("Input", input);
imshow("Output", output);
waitKey(0);
return 0;
}
```
注意,输入Mat对象必须是浮点数类型的,否则可能会导致结果不准确。同时,需要注意输入和输出Mat对象的大小和类型需要匹配。
相关问题
用c++ opencv实现mat的pow运算
在C++中,我们可以使用OpenCV库中的cv::pow函数来实现Mat的pow运算。该函数的原型如下:
```cpp
void cv::pow(InputArray src, double power, OutputArray dst);
```
其中,src是输入的Mat对象,power是幂指数,dst是输出的Mat对象。
下面是一个示例代码,实现对Mat对象的2次幂运算:
```cpp
#include <opencv2/core.hpp>
#include <opencv2/highgui.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main()
{
Mat input = imread("test.jpg", IMREAD_GRAYSCALE);
Mat output;
double power = 2.0;
cv::pow(input, power, output);
imshow("Input", input);
imshow("Output", output);
waitKey(0);
return 0;
}
```
注意,这里的幂指数必须为double类型。同时,需要注意输入和输出Mat对象的大小和类型需要匹配。
c++ opencv 创建Mat
Python OpenCV可以通过以下代码创建一个空的Mat对象:
```
import cv2
# 创建一个空的Mat对象
mat = cv2.Mat()
```
如果需要创建一个指定大小和类型的Mat对象,可以使用以下代码:
```
import cv2
# 创建一个3行4列,数据类型为float32的Mat对象
mat = cv2.Mat(3, 4, cv2.CV_32F)
```
其中,cv2.CV_32F表示数据类型为float32,可以根据需要选择不同的数据类型。
相关推荐
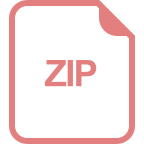
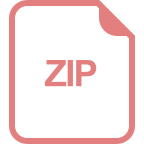
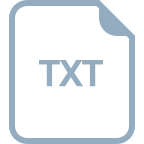
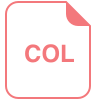
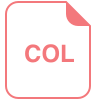
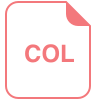
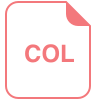
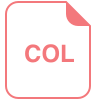







