堆栈实现算数表达式
时间: 2023-06-29 20:17:38 浏览: 100
堆栈可以用来实现算数表达式的计算。
具体实现步骤如下:
1. 创建一个操作数栈和一个操作符栈。
2. 从左到右遍历表达式,对于每个字符:
- 如果它是一个数字,则将其压入操作数栈。
- 如果它是一个操作符,则将其压入操作符栈。
- 如果它是一个右括号,则弹出操作符栈中的操作符和操作数栈中的操作数,直到遇到左括号为止。然后将计算结果压入操作数栈。
3. 最后,操作符栈中可能还有操作符,需要按照优先级顺序弹出操作符并计算,直到操作符栈为空。最后的操作数栈中的元素即为表达式的计算结果。
下面是一个示例代码,实现了一个简单的算数表达式计算器:
```python
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def calculate(expression):
precedence = {'+': 1, '-': 1, '*': 2, '/': 2, '(': 0, ')': 0}
operand_stack = Stack()
operator_stack = Stack()
for token in expression:
if token.isdigit():
operand_stack.push(int(token))
elif token in '+-*/':
while not operator_stack.is_empty() and precedence[token] <= precedence[operator_stack.peek()]:
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = eval(f'{operand1} {operator} {operand2}')
operand_stack.push(result)
operator_stack.push(token)
elif token == '(':
operator_stack.push(token)
elif token == ')':
while operator_stack.peek() != '(':
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = eval(f'{operand1} {operator} {operand2}')
operand_stack.push(result)
operator_stack.pop()
while not operator_stack.is_empty():
operator = operator_stack.pop()
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = eval(f'{operand1} {operator} {operand2}')
operand_stack.push(result)
return operand_stack.pop()
expression = '2 * (3 + 4) - 5 / 2'
result = calculate(expression)
print(f'{expression} = {result}')
```
输出结果为:
```
2 * (3 + 4) - 5 / 2 = 13.5
```
注意,这个实现只支持整数和四则运算,没有处理错误输入和除数为0的情况。在实际应用中,需要加入更多的判断和处理逻辑。
阅读全文
相关推荐

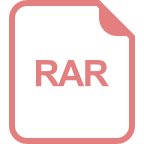


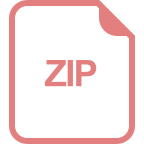
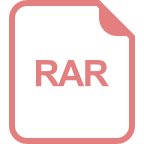
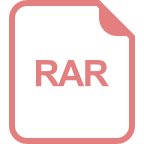
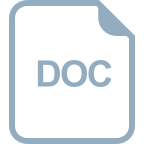
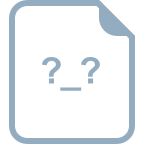
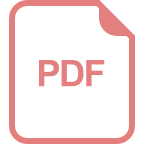
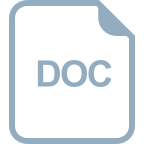
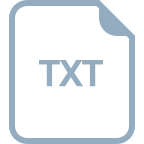
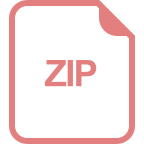
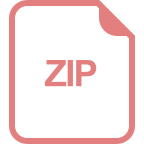
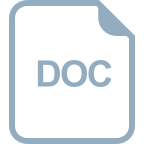