凯撒密码加密的C语言实现
时间: 2023-10-17 09:05:41 浏览: 152
以下是一个简单的凯撒密码加密的C语言实现:
```c
#include <stdio.h>
#include <string.h>
void caesar_encrypt(char *plaintext, int key) {
int i;
char ch;
for(i = 0; i < strlen(plaintext); ++i) {
ch = plaintext[i];
// Encrypt uppercase letters
if(ch >= 'A' && ch <= 'Z') {
ch = ch + key;
if(ch > 'Z') {
ch = ch - 'Z' + 'A' - 1;
}
plaintext[i] = ch;
}
// Encrypt lowercase letters
else if(ch >= 'a' && ch <= 'z') {
ch = ch + key;
if(ch > 'z') {
ch = ch - 'z' + 'a' - 1;
}
plaintext[i] = ch;
}
}
}
int main() {
char plaintext[100];
int key;
printf("Enter text to encrypt: ");
fgets(plaintext, 100, stdin);
printf("Enter key: ");
scanf("%d", &key);
caesar_encrypt(plaintext, key);
printf("Encrypted text: %s", plaintext);
return 0;
}
```
该程序首先从用户输入中获取明文和密钥,然后使用`caesar_encrypt()`函数对明文进行加密。该函数遍历明文中的每个字符,并根据其ASCII码值进行加密。如果字符是大写字母,则将其加上密钥,并根据需要将其“卷回”到字母表的开头。小写字母的加密过程类似。最后,程序输出加密后的文本。
阅读全文
相关推荐
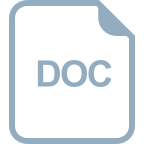
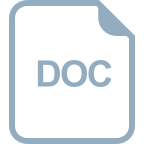
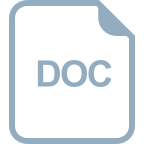
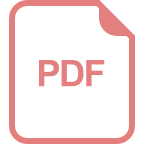
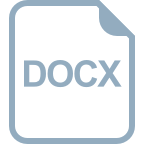
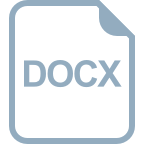
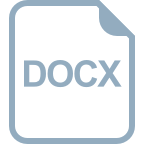
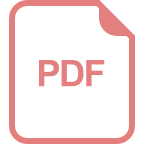
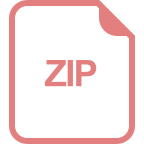
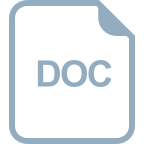
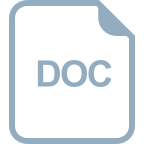
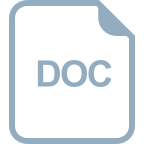
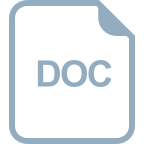
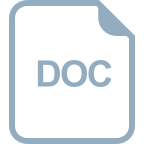


