OkHttp实现带进度条上传文件
时间: 2024-02-27 18:51:20 浏览: 30
在 OkHttp 中实现带进度条上传文件,可以使用 RequestBody 的子类 ProgressRequestBody 来实现,具体代码如下:
```
public class ProgressRequestBody extends RequestBody {
private RequestBody requestBody;
private UploadProgressListener progressListener;
public ProgressRequestBody(RequestBody requestBody, UploadProgressListener progressListener) {
this.requestBody = requestBody;
this.progressListener = progressListener;
}
@Override
public MediaType contentType() {
return requestBody.contentType();
}
@Override
public long contentLength() throws IOException {
return requestBody.contentLength();
}
@Override
public void writeTo(BufferedSink sink) throws IOException {
BufferedSink bufferedSink;
if (sink instanceof Buffer) {
bufferedSink = sink;
} else {
bufferedSink = Okio.buffer(sink);
}
CountingSink countingSink = new CountingSink(bufferedSink);
BufferedSink progressSink = Okio.buffer(countingSink);
requestBody.writeTo(progressSink);
progressSink.flush();
progressListener.onProgress(countingSink.getContentLength());
}
private class CountingSink extends ForwardingSink {
private long contentLength;
private long bytesWritten;
public CountingSink(Sink delegate) {
super(delegate);
contentLength = -1;
bytesWritten = 0;
}
@Override
public void write(Buffer source, long byteCount) throws IOException {
super.write(source, byteCount);
if (contentLength == -1) {
contentLength = contentLength();
}
bytesWritten += byteCount;
}
public long getContentLength() {
return contentLength;
}
public long getBytesWritten() {
return bytesWritten;
}
}
}
```
其中,UploadProgressListener 为上传进度监听器,代码如下:
```
public interface UploadProgressListener {
void onProgress(long bytesWritten);
}
```
在上传文件的时候,将 ProgressRequestBody 作为 RequestBody 使用即可:
```
OkHttpClient client = new OkHttpClient();
File file = new File("path/to/file");
RequestBody requestBody = new MultipartBody.Builder()
.setType(MultipartBody.FORM)
.addFormDataPart("file", file.getName(), new ProgressRequestBody(RequestBody.create(MediaType.parse("application/octet-stream"), file), new UploadProgressListener() {
@Override
public void onProgress(long bytesWritten) {
// 更新上传进度
}
}))
.build();
Request request = new Request.Builder()
.url("http://localhost/upload")
.post(requestBody)
.build();
Response response = client.newCall(request).execute();
```
在 ProgressRequestBody 中,每次写入数据的时候,都会回调 onProgress 方法,可以在这里更新上传进度。
相关推荐
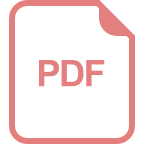
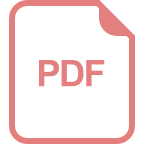
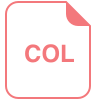
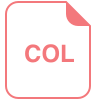
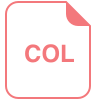
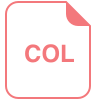
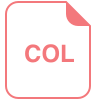









