constraintLayout.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // 获取列内容 String code = ((TextView) v.findViewById(R.id.code)).getText().toString(); String name = ((TextView) v.findViewById(R.id.name)).getText().toString(); String spec = ((TextView) v.findViewById(R.id.spec)).getText().toString(); String composition = ((TextView) v.findViewById(R.id.composition)).getText().toString(); String weight = ((TextView) v.findViewById(R.id.weight)).getText().toString(); // 创建弹窗布局 View popupView = getLayoutInflater().inflate(R.layout.showlist, null); // 获取弹窗布局中的控件 TextView titleView = popupView.findViewById(R.id.title); TextView contentView = popupView.findViewById(R.id.content); // 设置弹窗标题和内容 titleView.setText(name); contentView.setText("Code: " + code + "\n" + "Spec: " + spec + "\n" + "Composition: " + composition + "\n" + "Weight: " + weight); // 创建弹窗 PopupWindow popupWindow = new PopupWindow(popupView, WindowManager.LayoutParams.WRAP_CONTENT, WindowManager.LayoutParams.WRAP_CONTENT); // 设置弹窗的背景和动画 popupWindow.setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT)); popupWindow.setAnimationStyle(R.style.PopupAnimation); // 显示弹窗 popupWindow.showAtLocation(v, Gravity.CENTER, 0, 0); } });java.lang.NullPointerException: Attempt to invoke virtual method 'void androidx.constraintlayout.widget.ConstraintLayout.setOnClickListener(android.view.View$OnClickListener)' on a null object reference怎么解决
时间: 2024-02-14 15:08:17 浏览: 140
这个错误提示表明在调用 `setOnClickListener` 方法时,`ConstraintLayout` 对象为空,也就是没有被初始化。可以检查以下几个方面:
1. 确认 `ConstraintLayout` 是否在布局文件中被正确地定义和引用了。
2. 确认 `findViewById` 方法是否找到了正确的 `ConstraintLayout` 对象。
3. 确认代码中是否存在其他的逻辑错误,导致 `ConstraintLayout` 对象被释放或被置为 null。
你可以在调用 `setOnClickListener` 方法之前,加上以下代码段,来检查是否成功找到了 `ConstraintLayout` 对象:
```
if (constraintLayout == null) {
Log.e("TAG", "ConstraintLayout is null");
} else {
constraintLayout.setOnClickListener(new View.OnClickListener() {
// ...
});
}
```
相关问题
@Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); recyclerView = findViewById(R.id.recycler_view); button = findViewById(R.id.take_photo); //点击拍照 button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if(ContextCompat.checkSelfPermission(getApplicationContext(),Manifest.permission.CAMERA)!=PackageManager.PERMISSION_GRANTED){ ActivityCompat.requestPermissions(MainActivity.this,new String[]{Manifest.permission.CAMERA},REQUEST_CAMERA_PERMISSION); }else { takePicture(); } } }); }这个代码里面怎么添加保存照片的操作
你可以在 `takePicture()` 方法中添加保存照片的操作。具体来说,你可以在拍照成功后得到照片的 `Bitmap` 对象,然后将其保存到本地文件中。可以按照以下步骤操作:
1. 在 `onCreate()` 方法中添加文件存储权限的申请:
```java
if (ContextCompat.checkSelfPermission(getApplicationContext(), Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_STORAGE_PERMISSION);
}
```
2. 在 `takePicture()` 方法中获取拍照结果的 `Bitmap` 对象,并将其保存到文件中:
```java
private void takePicture() {
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (intent.resolveActivity(getPackageManager()) != null) {
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
ex.printStackTrace();
}
if (photoFile != null) {
Uri photoURI = FileProvider.getUriForFile(this, "com.example.android.fileprovider", photoFile);
intent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(intent, REQUEST_IMAGE_CAPTURE);
}
}
}
private File createImageFile() throws IOException {
// 创建一个以时间戳命名的文件名,防止文件名重复
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
// 获取系统默认的图片存储路径
File storageDir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES);
// 创建存储文件
File image = File.createTempFile(imageFileName, ".jpg", storageDir);
// 将存储文件的路径保存到全局变量中
mCurrentPhotoPath = image.getAbsolutePath();
return image;
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
// 获取拍照结果的Bitmap对象
Bitmap bitmap = BitmapFactory.decodeFile(mCurrentPhotoPath);
// 保存Bitmap到文件中
try {
FileOutputStream out = new FileOutputStream(mCurrentPhotoPath);
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, out);
out.flush();
out.close();
} catch (Exception e) {
e.printStackTrace();
}
// 显示照片
ImageView imageView = findViewById(R.id.imageView);
imageView.setImageBitmap(bitmap);
}
}
```
在上述代码中,`createImageFile()` 方法用于创建一个以时间戳命名的图片文件,`onActivityResult()` 方法用于获取拍照结果的 `Bitmap` 对象,并将其保存到文件中。保存时使用 `FileOutputStream` 将 `Bitmap` 写入文件中,并指定保存格式为 JPEG,压缩质量为 100。
在保存文件前,你需要先在 AndroidManifest.xml 文件中添加以下代码,以声明一个 FileProvider:
```xml
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="com.example.android.fileprovider"
android:grantUriPermissions="true"
android:exported="false">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
```
在 res/xml 目录下创建一个名为 file_paths.xml 的文件,内容如下:
```xml
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="pictures" path="Pictures" />
</paths>
```
上述代码中,`android:authorities` 属性需要指定一个唯一的字符串,以便其他应用程序可以获取你的应用程序的 FileProvider。在上述代码中,我们使用了 com.example.android.fileprovider 这个字符串。
注意:在使用 `FileProvider.getUriForFile()` 方法获取文件 Uri 时,第一个参数需要传递一个 Context 对象。如果你在 Activity 中使用该方法,可以直接使用 `this` 关键字;如果在 Fragment 中使用该方法,需要使用 `getActivity()` 获取 Activity 对象。
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_estimate); Intent intent = getIntent(); String code = intent.getStringExtra("code"); String name = intent.getStringExtra("name"); final long ranking = intent.getLongExtra("ranking",0L); final float pe = intent.getFloatExtra("pe", 0.0f); final float pb = intent.getFloatExtra("pb", 0.0f); tv_code = (TextView) findViewById(R.id.tv_code); tv_name = (TextView) findViewById(R.id.tv_name); tv_ranking = (TextView) findViewById(R.id.tv_ranking); tv_pe = (TextView) findViewById(R.id.tv_pe); tv_pb = (TextView) findViewById(R.id.tv_pb); tv_suggestEstimate = (TextView) findViewById(R.id.tv_suggestEstimate); tv_code.setText("姓名:" + code); tv_name.setText("单位:" + name); tv_ranking.setText("手机号码:" + ranking); tv_pe.setText("邮件:" + pe); tv_pb.setText("备注:" + pb); btn_estimate = (Button) findViewById(R.id.btn_estimate); btn_estimate.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { estimateResult = estimateData(ranking, pe, pb); //Log.i("EA", estimateResult) ; tv_suggestEstimate.setText("提示:" + estimateResult); } }); btn_return = (Button) findViewById(R.id.btn_return); btn_return.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(); intent.putExtra("data", estimateResult); setResult(2, intent); finish(); } }); }如何去掉final
如果想要去掉 `final` 关键字,需要注意以下几点:
1. `ranking`、`pe`、`pb` 这三个变量在 `onClick` 方法中被使用,如果去掉 `final`,则这三个变量如果在 `onClick` 方法中被修改,编译时会报错。
2. 如果想要去掉 `final`,则需要将这三个变量定义为 Activity 的成员变量,而不是在 `onCreate` 方法中定义。
下面是修改后的代码:
```java
private long ranking;
private float pe;
private float pb;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_estimate);
Intent intent = getIntent();
String code = intent.getStringExtra("code");
String name = intent.getStringExtra("name");
ranking = intent.getLongExtra("ranking",0L);
pe = intent.getFloatExtra("pe", 0.0f);
pb = intent.getFloatExtra("pb", 0.0f);
tv_code = (TextView) findViewById(R.id.tv_code);
tv_name = (TextView) findViewById(R.id.tv_name);
tv_ranking = (TextView) findViewById(R.id.tv_ranking);
tv_pe = (TextView) findViewById(R.id.tv_pe);
tv_pb = (TextView) findViewById(R.id.tv_pb);
tv_suggestEstimate = (TextView) findViewById(R.id.tv_suggestEstimate);
tv_code.setText("姓名:" + code);
tv_name.setText("单位:" + name);
tv_ranking.setText("手机号码:" + ranking);
tv_pe.setText("邮件:" + pe);
tv_pb.setText("备注:" + pb);
btn_estimate = (Button) findViewById(R.id.btn_estimate);
btn_estimate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
estimateResult = estimateData(ranking, pe, pb);
//Log.i("EA", estimateResult) ;
tv_suggestEstimate.setText("提示:" + estimateResult);
}
});
btn_return = (Button) findViewById(R.id.btn_return);
btn_return.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent();
intent.putExtra("data", estimateResult);
setResult(2, intent);
finish();
}
});
}
```
阅读全文
相关推荐
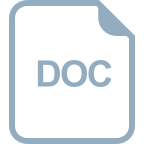
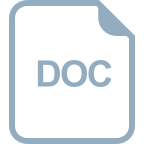
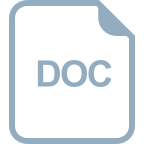













