写一段使用Grahpics.Blit的绘画代码
时间: 2024-03-27 20:37:34 浏览: 24
以下是一个使用 `Graphics.Blit` 绘制简单图形的示例代码:
```csharp
using UnityEngine;
public class PaintExample : MonoBehaviour
{
public Material paintMaterial;
public Texture2D brushTexture;
public Color brushColor = Color.white;
public float brushSize = 0.1f;
private RenderTexture renderTexture;
void Start()
{
// 创建渲染纹理
renderTexture = new RenderTexture(512, 512, 0, RenderTextureFormat.ARGB32);
renderTexture.Create();
}
void Update()
{
// 当用户按下鼠标左键时,开始绘画
if (Input.GetMouseButton(0))
{
// 计算画笔位置和大小
Vector2 mousePosition = Input.mousePosition;
mousePosition.y = Screen.height - mousePosition.y;
float pixelSize = brushSize * (float)Screen.height / (float)renderTexture.height;
// 在渲染纹理上绘制画笔
RenderTexture.active = renderTexture;
paintMaterial.SetTexture("_BrushTex", brushTexture);
paintMaterial.SetColor("_BrushColor", brushColor);
paintMaterial.SetFloat("_BrushSize", pixelSize);
paintMaterial.SetFloat("_MouseX", mousePosition.x / (float)Screen.width);
paintMaterial.SetFloat("_MouseY", mousePosition.y / (float)Screen.height);
paintMaterial.SetPass(0);
Graphics.Blit(renderTexture, renderTexture);
// 将渲染纹理绘制到屏幕上
RenderTexture.active = null;
Graphics.Blit(renderTexture, null);
}
}
}
```
在上面的示例中,我们创建了一个渲染纹理,并在用户按下鼠标左键时,在渲染纹理上绘制了一个圆形的画笔。我们使用 `RenderTexture.active` 属性将渲染纹理设置为当前渲染目标,然后使用 `Graphics.Blit` 函数将渲染纹理传递给着色器进行绘制。最后,我们使用 `RenderTexture.active = null` 将渲染目标设置回屏幕,并使用 `Graphics.Blit` 函数将渲染纹理绘制到屏幕上。
要使此示例正常工作,您需要创建一个材质和纹理,以用于绘制画笔。在材质中,您需要编写一个着色器来绘制画笔,例如使用纹理和 alpha 值进行混合。
相关推荐
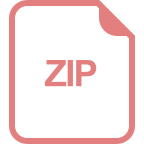
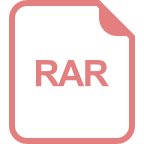
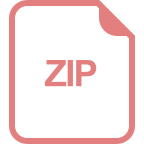

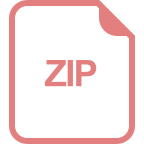
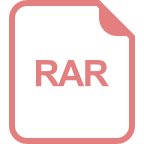
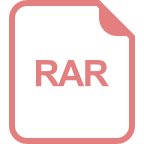
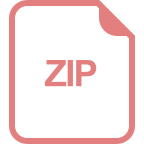
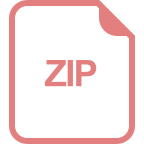
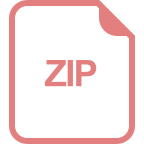
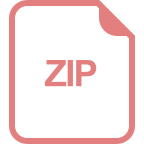
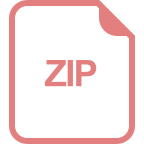
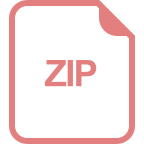