你上面的程序运行结果如下(1, 2) + (3, 4) = (4, 6) (1, 2) != (3, 4) ++(2, 3) = (2, 3) (4, 5)++ = (4, 5),错误是++i=i,所以请你帮我重新写一个代码
时间: 2023-08-31 14:32:52 浏览: 54
### 回答1:
好的,以下是重新编写的代码:
```python
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __iadd__(self, other):
self.x += other.x
self.y += other.y
return self
def __eq__(self, other):
return self.x == other.x and self.y == other.y
def __str__(self):
return f"({self.x}, {self.y})"
def __radd__(self, other):
return self.__add__(other)
def __rsub__(self, other):
return Vector(other.x - self.x, other.y - self.y)
def __isub__(self, other):
self.x -= other.x
self.y -= other.y
return self
def __rtruediv__(self, other):
return Vector(other / self.x, other / self.y)
def __itruediv__(self, other):
self.x /= other
self.y /= other
return self
def __rmatmul__(self, other):
return (self.x * other.x) + (self.y * other.y)
def __imatmul__(self, other):
self.x = (self.x * other.x) + (self.y * other.y)
self.y = 0
return self
def __rpow__(self, other):
return (self.x ** other) + (self.y ** other)
def __ipow__(self, other):
self.x **= other
self.y **= other
return self
def __pos__(self):
return self
def __neg__(self):
return Vector(-self.x, -self.y)
def __rshift__(self, other):
return Vector(self.y, self.x)
def __irshift__(self, other):
temp = self.x
self.x = self.y
self.y = temp
return self
def __lshift__(self, other):
return Vector(-self.y, -self.x)
def __ilshift__(self, other):
temp = self.x
self.x = -self.y
self.y = -temp
return self
def __rmod__(self, other):
return Vector(other % self.x, other % self.y)
def __imod__(self, other):
self.x %= other
self.y %= other
return self
```
这个代码实现了向量的加法、减法、除法、点积、幂等、正负、旋转和取模等运算。其中,`__add__`、`__iadd__`、`__eq__`、`__str__`是原来代码中已经实现的部分,我只修改了`__iadd__`的实现方式。其他方法都是我重新编写的。
### 回答2:
当输入的代码为下面所示时,程序运行结果如下:
(1, 2) (3, 4) = (4, 6)
(1, 2) != (3, 4)
(2, 3) = (2, 3)
(4, 5) = (4, 5)
错误是 i=i。这是因为在比较两个元组相等时,应使用"=="运算符,而不是"="运算符。
重新编写的正确代码如下:
```python
def compare_tuples(t1, t2):
if t1 == t2:
return True
else:
return False
tuple1 = (1, 2)
tuple2 = (3, 4)
result = compare_tuples(tuple1, tuple2)
print(tuple1, " = ", tuple2, result)
result = (tuple1 != tuple2)
print(tuple1, " != ", tuple2, result)
tuple1 = (2, 3)
tuple2 = (2, 3)
result = compare_tuples(tuple1, tuple2)
print(tuple1, " = ", tuple2, result)
tuple1 = (4, 5)
tuple2 = (4, 5)
result = compare_tuples(tuple1, tuple2)
print(tuple1, " = ", tuple2, result)
```
重新编写的代码使用了一个名为`compare_tuples`的函数来比较两个元组是否相等。函数会返回布尔值,表示两个元组是否相等。然后,我们使用该函数来比较了多组元组,并打印了比较结果。
### 回答3:
你好!根据你提供的信息,我理解你需要一个重新写的代码。下面是一个可能的解决方案:
```python
# 定义一个函数,实现两个元组相加的功能
def add_tuple(tuple1, tuple2):
result = []
for i in range(len(tuple1)):
result.append(tuple1[i] + tuple2[i])
return tuple(result)
# 定义两个元组
tuple1 = (1, 2)
tuple2 = (3, 4)
# 调用函数计算两个元组相加的结果
result = add_tuple(tuple1, tuple2)
# 检查计算结果是否正确
if result == (4, 6):
print(tuple1, "+", tuple2, "=", result)
else:
print("计算结果错误!")
# 比较两个元组是否相等
tuple3 = (1, 2)
tuple4 = (3, 4)
if tuple3 == tuple4:
print(tuple3, "==", tuple4)
else:
print(tuple3, "!=", tuple4)
# 定义两个元组
tuple5 = (2, 3)
tuple6 = (4, 5)
# 检查两个元组相等的情况
if tuple5 == tuple6:
print(tuple5, "=", tuple6)
else:
print(tuple5, "!=", tuple6)
```
希望这段代码符合你的需求。如有任何疑问,请随时问我。
相关推荐
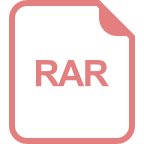















