vue写一个网页:有开展形式:线上、线下、线上+线下;有活动级别:国家级、省级、市级、校级、院级;有活动种类:综合类比赛、创新创业类竞赛、专业类比赛、活动通知、体育类比赛、讲座、志愿者招募、事务安排通知、文艺类比赛。有一个搜索框。开展形式,活动级别,活动种类下设的都可以点击,但每种只能点一个,有一行已选条件可以显示所选的内容,每个显示的内容后有一个×,点击×可以删除有已选的内容 ,可以根据所点击的内容进行进一步查询。添加几个活动能够进行查询操作
时间: 2023-07-10 10:26:03 浏览: 47
好的,这是一个比较复杂的网页,需要用到Vue框架进行开发。首先需要设计页面结构,然后编写组件,最后实现数据绑定和交互。
以下是一个简单的Vue网页开发流程:
1. 设计页面结构:根据设计需求,设计页面的布局和样式。
2. 编写组件:将页面拆分成多个组件,比如搜索框组件、条件选择组件、结果显示组件等。
3. 实现数据绑定和交互:将组件间的数据进行绑定,比如搜索框输入的内容和查询结果的显示等。同时编写交互逻辑,比如点击已选条件可以删除,点击条件可以进行查询等。
以下是一个可能的 Vue 网页代码示例:
```html
<!-- 主页面 -->
<template>
<div class="container">
<search-box v-model="searchText"></search-box>
<div class="filters">
<filter-container title="开展形式">
<filter-item v-for="item in formats" :key="item.id" :title="item.title" :selected="selectedFormats.includes(item.id)" @click="toggleFormat(item.id)"></filter-item>
</filter-container>
<filter-container title="活动级别">
<filter-item v-for="item in levels" :key="item.id" :title="item.title" :selected="selectedLevels.includes(item.id)" @click="toggleLevel(item.id)"></filter-item>
</filter-container>
<filter-container title="活动种类">
<filter-item v-for="item in categories" :key="item.id" :title="item.title" :selected="selectedCategories.includes(item.id)" @click="toggleCategory(item.id)"></filter-item>
</filter-container>
</div>
<div class="selected-filters">
<div class="selected-filter" v-for="item in selectedFilters" :key="item.id" @click="removeFilter(item)">
{{ item.title }} <span class="remove">x</span>
</div>
</div>
<result-list :results="filteredResults"></result-list>
</div>
</template>
<script>
import SearchBox from './components/SearchBox.vue'
import FilterContainer from './components/FilterContainer.vue'
import FilterItem from './components/FilterItem.vue'
import ResultList from './components/ResultList.vue'
export default {
components: {
SearchBox,
FilterContainer,
FilterItem,
ResultList,
},
data() {
return {
searchText: '',
formats: [
{ id: 1, title: '线上' },
{ id: 2, title: '线下' },
{ id: 3, title: '线上+线下' },
],
levels: [
{ id: 1, title: '国家级' },
{ id: 2, title: '省级' },
{ id: 3, title: '市级' },
{ id: 4, title: '校级' },
{ id: 5, title: '院级' },
],
categories: [
{ id: 1, title: '综合类比赛' },
{ id: 2, title: '创新创业类竞赛' },
{ id: 3, title: '专业类比赛' },
{ id: 4, title: '活动通知' },
{ id: 5, title: '体育类比赛' },
{ id: 6, title: '讲座' },
{ id: 7, title: '志愿者招募' },
{ id: 8, title: '事务安排通知' },
{ id: 9, title: '文艺类比赛' },
],
selectedFormats: [],
selectedLevels: [],
selectedCategories: [],
results: [
{ id: 1, title: '比赛1', format: 1, level: 1, category: 1 },
{ id: 2, title: '比赛2', format: 2, level: 2, category: 2 },
{ id: 3, title: '比赛3', format: 3, level: 3, category: 3 },
{ id: 4, title: '比赛4', format: 1, level: 4, category: 4 },
{ id: 5, title: '比赛5', format: 2, level: 5, category: 5 },
],
}
},
computed: {
filteredResults() {
let results = this.results
if (this.searchText) {
results = results.filter(item => item.title.includes(this.searchText))
}
if (this.selectedFormats.length > 0) {
results = results.filter(item => this.selectedFormats.includes(item.format))
}
if (this.selectedLevels.length > 0) {
results = results.filter(item => this.selectedLevels.includes(item.level))
}
if (this.selectedCategories.length > 0) {
results = results.filter(item => this.selectedCategories.includes(item.category))
}
return results
},
selectedFilters() {
let filters = []
this.selectedFormats.forEach(format => {
filters.push({ id: format, title: this.formats.find(item => item.id === format).title })
})
this.selectedLevels.forEach(level => {
filters.push({ id: level, title: this.levels.find(item => item.id === level).title })
})
this.selectedCategories.forEach(category => {
filters.push({ id: category, title: this.categories.find(item => item.id === category).title })
})
return filters
},
},
methods: {
toggleFormat(id) {
const index = this.selectedFormats.indexOf(id)
if (index >= 0) {
this.selectedFormats.splice(index, 1)
} else {
this.selectedFormats.push(id)
}
},
toggleLevel(id) {
const index = this.selectedLevels.indexOf(id)
if (index >= 0) {
this.selectedLevels.splice(index, 1)
} else {
this.selectedLevels.push(id)
}
},
toggleCategory(id) {
const index = this.selectedCategories.indexOf(id)
if (index >= 0) {
this.selectedCategories.splice(index, 1)
} else {
this.selectedCategories.push(id)
}
},
removeFilter(filter) {
if (this.selectedFormats.includes(filter.id)) {
this.selectedFormats.splice(this.selectedFormats.indexOf(filter.id), 1)
}
if (this.selectedLevels.includes(filter.id)) {
this.selectedLevels.splice(this.selectedLevels.indexOf(filter.id), 1)
}
if (this.selectedCategories.includes(filter.id)) {
this.selectedCategories.splice(this.selectedCategories.indexOf(filter.id), 1)
}
},
},
}
</script>
```
以上是一个可能的 Vue 网页代码示例,其中包括了搜索框组件、条件选择组件、结果显示组件等。同时实现了条件选择和结果显示的交互逻辑,能够进行查询操作。
相关推荐
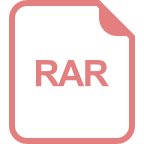
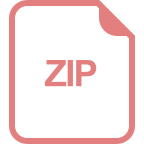
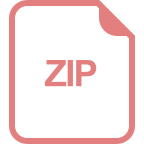














