oauth2实现短信验证码登录
时间: 2023-02-09 18:46:57 浏览: 137
好的。
OAuth 2.0 是一个开放的授权框架,它允许用户授权第三方应用访问他们的资源,而无需将用户名和密码提供给第三方应用。
在短信验证码登录中,首先用户需要向第三方应用发送一个请求,要求发送短信验证码到其手机。第三方应用收到请求后,会向用户手机发送一条包含验证码的短信。用户输入收到的验证码后,第三方应用会再次发送请求到服务器,验证验证码是否正确。如果验证码正确,服务器会给用户发放一个令牌(token),表示用户已经授权第三方应用访问其资源。之后,第三方应用就可以使用这个令牌访问用户的资源了。
总的来说,OAuth 2.0 可以帮助你实现短信验证码登录功能,但是你还需要一些其他的技术(例如发送短信和验证验证码)才能完成整个过程。
相关问题
Spring Security Oauth2.0 实现短信验证码登录
您好!要实现Spring Security OAuth2.0的短信验证码登录,您可以按照以下步骤操作:
1. 添加依赖:在您的项目中添加Spring Security和Spring Security OAuth2的依赖。
2. 创建用户表和验证码表:创建一个用户表用于存储用户信息,另外创建一个验证码表用于存储手机号、验证码和过期时间等信息。
3. 创建自定义的UserDetailsService:实现UserDetailsService接口,用于加载用户信息。这里需要根据手机号查询用户信息。
4. 创建自定义的AuthenticationProvider:实现AuthenticationProvider接口,于验证用户凭证。在这里可以验证手机号和验证码是否匹配。
5. 配置Spring Security:在Spring Security的配置文件中,配置AuthenticationProvider和UserDetailsService。
6. 创建自定义的Filter:继承AbstractAuthenticationProcessingFilter类,重写attemptAuthentication方法,用于处理短信验证码登录请求。
7. 创建自定义的Token生成器:实现TokenGranter接口,并在其中生成Token。
8. 配置OAuth2:在OAuth2配置文件中,配置TokenGranter和AuthenticationManager。
9. 配置短信验证码登录的接口:设置一个接口用于发送短信验证码,并在接口中调用自定义的Filter进行验证。
以上是一个基本的流程,您可以根据自己的需求进行适当的调整和扩展。希望对您有所帮助!如果有任何问题,请随时提问。
spring oauth2 短信验证码登录
Spring OAuth2提供了一个框架,可以使我们很容易地实现短信验证码登录。下面是实现该功能的简单步骤:
1. 添加依赖
在pom.xml文件中添加以下依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
```
2. 配置Security
在Spring Security配置文件中添加以下配置:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/oauth/token").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
}
```
这将启用Web安全性,并配置Spring Security以使用我们提供的用户详细信息服务和密码编码器。它还允许所有人访问OAuth2令牌端点(/oauth/token)。
3. 实现用户详细信息服务
我们需要实现UserDetailsService接口来从我们的数据库或其他数据源中获取用户详细信息。以下是一个示例:
```
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if(user == null){
throw new UsernameNotFoundException("User not found with username: " + username);
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(),
new ArrayList<>());
}
}
```
此服务返回Spring Security的UserDetails对象,该对象包含有关用户的详细信息。
4. 实现自定义的短信验证码登录过滤器
我们需要实现一个自定义的过滤器来处理短信验证码登录。以下是一个示例:
```
public class SmsCodeAuthenticationFilter extends AbstractAuthenticationProcessingFilter {
public static final String SPRING_SECURITY_FORM_MOBILE_KEY = "mobile";
public static final String SPRING_SECURITY_FORM_CODE_KEY = "code";
private String mobileParameter = SPRING_SECURITY_FORM_MOBILE_KEY;
private String codeParameter = SPRING_SECURITY_FORM_CODE_KEY;
private boolean postOnly = true;
public SmsCodeAuthenticationFilter() {
super(new AntPathRequestMatcher("/login/mobile", "POST"));
}
@Override
public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response)
throws AuthenticationException, IOException, ServletException {
if (postOnly && !request.getMethod().equals("POST")) {
throw new AuthenticationServiceException(
"Authentication method not supported: " + request.getMethod());
}
String mobile = obtainMobile(request);
String code = obtainCode(request);
if (mobile == null) {
mobile = "";
}
if (code == null) {
code = "";
}
mobile = mobile.trim();
SmsCodeAuthenticationToken authRequest = new SmsCodeAuthenticationToken(mobile, code);
setDetails(request, authRequest);
return this.getAuthenticationManager().authenticate(authRequest);
}
protected String obtainMobile(HttpServletRequest request) {
return request.getParameter(mobileParameter);
}
protected String obtainCode(HttpServletRequest request) {
return request.getParameter(codeParameter);
}
protected void setDetails(HttpServletRequest request, SmsCodeAuthenticationToken authRequest) {
authRequest.setDetails(authenticationDetailsSource.buildDetails(request));
}
public void setMobileParameter(String mobileParameter) {
Assert.hasText(mobileParameter, "Mobile parameter must not be empty or null");
this.mobileParameter = mobileParameter;
}
public void setPostOnly(boolean postOnly) {
this.postOnly = postOnly;
}
public final String getMobileParameter() {
return mobileParameter;
}
public String getCodeParameter() {
return codeParameter;
}
public void setCodeParameter(String codeParameter) {
Assert.hasText(codeParameter, "Code parameter must not be empty or null");
this.codeParameter = codeParameter;
}
}
```
此过滤器将处理POST请求,其中包含用户的手机号码和短信验证码。它将创建一个SmsCodeAuthenticationToken对象,该对象将包含有关用户的信息。在这里,我们还可以设置其他详细信息,例如IP地址和请求的时间戳等。
5. 配置认证管理器
在Spring Security配置文件中添加以下配置:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private SmsCodeAuthenticationProvider smsCodeAuthenticationProvider;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
auth.authenticationProvider(smsCodeAuthenticationProvider);
}
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/oauth/token").permitAll()
.antMatchers("/login/mobile").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
http.addFilterBefore(smsCodeAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
@Bean
public SmsCodeAuthenticationFilter smsCodeAuthenticationFilter() throws Exception {
SmsCodeAuthenticationFilter filter = new SmsCodeAuthenticationFilter();
filter.setAuthenticationManager(authenticationManagerBean());
return filter;
}
@Bean
public SmsCodeAuthenticationProvider smsCodeAuthenticationProvider(){
return new SmsCodeAuthenticationProvider();
}
@Bean
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
}
```
这将配置我们的认证管理器,并告诉Spring Security使用我们自定义的SmsCodeAuthenticationProvider来验证短信验证码。我们还需要将自定义的SmsCodeAuthenticationFilter添加到过滤器链中。
6. 实现自定义的短信验证码认证提供程序
我们需要实现一个自定义的认证提供程序来验证短信验证码。以下是一个示例:
```
public class SmsCodeAuthenticationProvider implements AuthenticationProvider {
@Autowired
private UserDetailsService userDetailsService;
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
SmsCodeAuthenticationToken authenticationToken = (SmsCodeAuthenticationToken) authentication;
UserDetails userDetails = userDetailsService.loadUserByUsername((String) authenticationToken.getPrincipal());
if(userDetails == null){
throw new InternalAuthenticationServiceException("无法获取用户信息");
}
SmsCode smsCode = (SmsCode) authenticationToken.getCredentials();
if(!smsCode.getCode().equals("123456")){
throw new BadCredentialsException("短信验证码不正确");
}
SmsCodeAuthenticationToken authenticationResult = new SmsCodeAuthenticationToken(userDetails, userDetails.getAuthorities());
authenticationResult.setDetails(authenticationToken.getDetails());
return authenticationResult;
}
@Override
public boolean supports(Class<?> authentication) {
return SmsCodeAuthenticationToken.class.isAssignableFrom(authentication);
}
}
```
此提供程序将验证提供的短信验证码,并返回一个已验证的SmsCodeAuthenticationToken对象。
最后,我们就可以使用短信验证码登录了。用户只需在登录页面上输入其手机号码和收到的短信验证码即可成功登录。
阅读全文
相关推荐
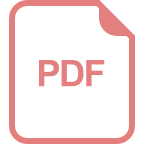
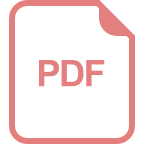
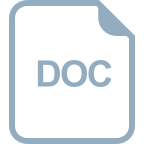

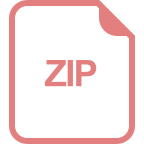
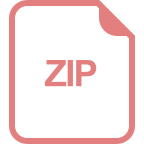
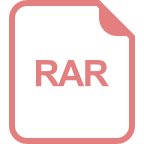
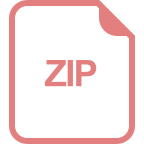
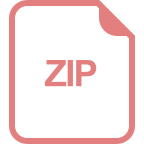
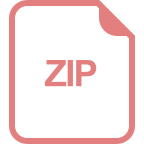
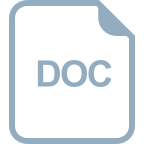
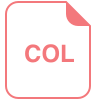
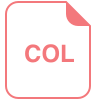
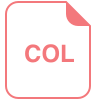

