lcd触摸拖动的c语言代码实现
时间: 2023-05-30 10:06:46 浏览: 140
以下是一个基本的LCD触摸拖动的C语言代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/input.h>
#include <linux/fb.h>
#include <sys/mman.h>
#define FBDEVNAME "/dev/fb0"
#define EVENTNAME "/dev/input/event0"
int main(void)
{
int fbfd;
struct fb_var_screeninfo vinfo;
struct fb_fix_screeninfo finfo;
char *fbp = 0;
int x, y, dx, dy;
int prev_x = 0, prev_y = 0, curr_x = 0, curr_y = 0;
int touch_down = 0, touch_up = 1;
int evfd;
struct input_event ev;
fbfd = open(FBDEVNAME, O_RDWR);
if (!fbfd) {
printf("Error: cannot open framebuffer device.\n");
exit(1);
}
if (ioctl(fbfd, FBIOGET_FSCREENINFO, &finfo)) {
printf("Error: reading fixed information.\n");
exit(2);
}
if (ioctl(fbfd, FBIOGET_VSCREENINFO, &vinfo)) {
printf("Error: reading variable information.\n");
exit(3);
}
fbp = (char *)mmap(0, finfo.smem_len, PROT_READ | PROT_WRITE, MAP_SHARED, fbfd, 0);
if ((int)fbp == -1) {
printf("Error: failed to map framebuffer device to memory.\n");
exit(4);
}
evfd = open(EVENTNAME, O_RDONLY);
if (!evfd) {
printf("Error: cannot open input device.\n");
exit(1);
}
while (1) {
if (read(evfd, &ev, sizeof(struct input_event)) < sizeof(struct input_event)) {
printf("Error: failed to read input event.\n");
exit(1);
}
if (ev.type == EV_ABS) {
if (ev.code == ABS_X) {
curr_x = ev.value;
} else if (ev.code == ABS_Y) {
curr_y = ev.value;
} else if (ev.code == ABS_MT_POSITION_X) {
curr_x = ev.value;
} else if (ev.code == ABS_MT_POSITION_Y) {
curr_y = ev.value;
} else if (ev.code == ABS_MT_TRACKING_ID) {
if (ev.value == -1) {
touch_down = 0;
touch_up = 1;
} else {
touch_down = 1;
touch_up = 0;
}
}
} else if (ev.type == EV_SYN && touch_down && !touch_up) {
dx = curr_x - prev_x;
dy = curr_y - prev_y;
x += dx;
y += dy;
if (x < 0) {
x = 0;
}
if (x > vinfo.xres - 1) {
x = vinfo.xres - 1;
}
if (y < 0) {
y = 0;
}
if (y > vinfo.yres - 1) {
y = vinfo.yres - 1;
}
*((unsigned short*)(fbp + (x + y * vinfo.xres) * 2)) = 0xFFFF;
prev_x = curr_x;
prev_y = curr_y;
}
}
munmap(fbp, finfo.smem_len);
close(fbfd);
close(evfd);
return 0;
}
```
该代码使用了Linux的输入系统和帧缓冲系统来实现LCD触摸拖动功能。它使用了evdev驱动程序来读取触摸屏幕输入事件,并将触摸坐标映射到帧缓冲内存中的像素坐标。最后,它在指定的像素坐标处绘制了一个白色的像素点。
阅读全文
相关推荐


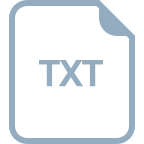
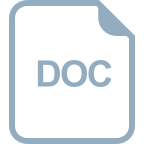
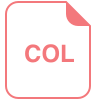
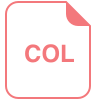
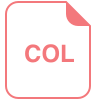


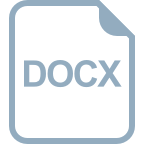