分析以下程序,会有僵尸进程出现吗?后台运行该程序,前台用ps命令查看进程,判断是否出现僵尸进程,如果出现,请用三种方法(wait/waitpid,两次fork,捕捉信号SIGCHLD)修改程序处理僵尸进程。#include<stdio.h> #include<stdlib.h> #include<sys/types.h> #include<sys/wait.h> main() {int pid; if ((pid=fork())==0) { sleep(2);printf("child process %d exit\n",getpid());exit(0);} else { printf("hello child\n"); while(1){ sleep(1); }; } }
时间: 2024-01-16 19:02:38 浏览: 114
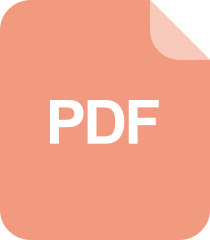
ps命令输出进程状态S+的含义解析
这个程序可能会出现僵尸进程,因为父进程没有等待子进程的结束,子进程结束后会成为僵尸进程。
下面是三种修改程序处理僵尸进程的方法:
1. 使用wait/waitpid函数:
```
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
int pid;
if ((pid = fork()) == 0) {
sleep(2);
printf("child process %d exit\n", getpid());
exit(0);
} else {
printf("hello child\n");
wait(NULL); // 等待子进程结束
while (1) {
sleep(1);
}
}
return 0;
}
```
2. 使用两次fork函数:
```
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
int pid;
if ((pid = fork()) == 0) {
if ((pid = fork()) == 0) {
sleep(2);
printf("grandson process %d exit\n", getpid());
exit(0);
} else {
exit(0);
}
} else {
printf("hello child\n");
wait(NULL); // 等待子进程结束
while (1) {
sleep(1);
}
}
return 0;
}
```
3. 捕捉信号SIGCHLD:
```
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <signal.h>
void sig_handler(int signo) {
if (signo == SIGCHLD) {
wait(NULL);
}
}
int main() {
int pid;
signal(SIGCHLD, sig_handler); // 捕捉信号SIGCHLD
if ((pid = fork()) == 0) {
sleep(2);
printf("child process %d exit\n", getpid());
exit(0);
} else {
printf("hello child\n");
while (1) {
sleep(1);
}
}
return 0;
}
```
阅读全文
相关推荐
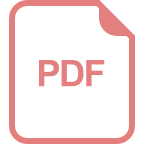
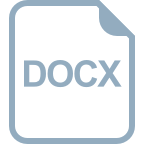














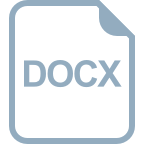
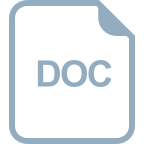