帮我写一段spring aop的代码
时间: 2023-11-03 15:11:06 浏览: 103
好的,以下是一段简单的Spring AOP代码:
1. 首先,我们需要定义一个切面类,用于实现AOP的逻辑。
```java
package com.example.demo.aop;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
private static final Logger logger = LoggerFactory.getLogger(LoggingAspect.class);
@Before("execution(* com.example.demo.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
logger.info("Before method " + joinPoint.getSignature().getName() + " is called.");
}
@AfterReturning(pointcut = "execution(* com.example.demo.service.*.*(..))", returning = "result")
public void logAfter(JoinPoint joinPoint, Object result) {
logger.info("After method " + joinPoint.getSignature().getName() + " is called. Result is " + result);
}
}
```
2. 在上面的代码中,我们使用了@Aspect注释来标识这是一个切面类,并使用@Before和@AfterReturning注释来定义切入点和AOP逻辑。具体来说:
- 在@Before注释中,我们使用了一个execution表达式来定义切入点,它表示在任何一个com.example.demo.service包下的任何方法被调用之前都会执行logBefore方法。
- 在@AfterReturning注释中,我们同样使用了一个execution表达式来定义切入点,并指定了返回值类型为Object。这意味着只有在com.example.demo.service包下的任何方法被调用并返回一个非null值时,才会执行logAfter方法。
3. 最后,我们需要在Spring配置文件中配置该切面类。
```xml
<bean id="loggingAspect" class="com.example.demo.aop.LoggingAspect"/>
<aop:config>
<aop:aspect ref="loggingAspect">
<aop:before pointcut="execution(* com.example.demo.service.*.*(..))" method="logBefore"/>
<aop:after-returning pointcut="execution(* com.example.demo.service.*.*(..))" returning="result" method="logAfter"/>
</aop:aspect>
</aop:config>
```
在上面的配置中,我们首先定义了一个loggingAspect bean,然后使用<aop:config>标签来定义整个AOP配置。在<aop:aspect>标签中,我们指定了切面类的引用,并使用<aop:before>和<aop:after-returning>标签来定义切入点和AOP逻辑。注意,这些标签中的pointcut和method属性分别对应切面类中的@Before和@AfterReturning注释中的值。
阅读全文
相关推荐





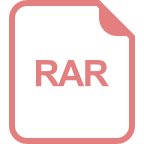







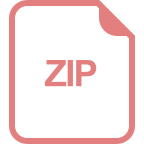
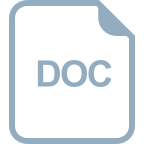
