编写c语言函数求给定字符串中数字字符个数
时间: 2024-05-02 11:23:40 浏览: 14
好的,我明白了。下面是求给定字符串中数字字符个数的C语言函数:
```c
#include <stdio.h>
#include <ctype.h>
int count_digits(char* str) {
int count = 0;
while (*str) {
if (isdigit(*str)) {
count++;
}
str++;
}
return count;
}
int main() {
char str[] = "a12c3d456f";
int count = count_digits(str);
printf("数字字符个数:%d\n", count);
return 0;
}
```
运行结果:
```
数字字符个数:6
```
请问有没问题?
相关问题
利用C语言,编写函数,求给定字符串中数字字符的个数
好的,这个问题可以回答。下面是代码:
```c
#include <stdio.h>
#include <ctype.h>
int count_digits(char* str) {
int count = 0;
while (*str != '\0') {
if (isdigit(*str)) {
count++;
}
str++;
}
return count;
}
int main() {
char* str = "abc123def456";
int num_digits = count_digits(str);
printf("Number of digits: %d\n", num_digits);
return 0;
}
```
这个程序通过循环遍历字符串中的每一个字符,使用 `isdigit` 函数来判断是否是数字字符,如果是则计数器加一。最后返回计数器的值,即数字字符的个数。
编写C语言,求给定字符串中数字字符的个数,在主函数中输入及输出统计的个数。
以下是一个简单的C语言程序,可以实现统计给定字符串中数字字符的个数:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
int count = 0;
printf("请输入一个字符串:");
scanf("%s", str);
for (int i = 0; i < strlen(str); i++) {
if (str[i] >= '0' && str[i] <= '9') {
count++;
}
}
printf("字符串中数字字符的个数为:%d\n", count);
return 0;
}
```
程序首先定义了一个字符串变量 `str`,用于存储输入的字符串,以及一个整型变量 `count`,用于统计数字字符的个数。然后通过 `scanf` 函数从标准输入中读取一个字符串,并通过 `strlen` 函数获取该字符串的长度。接着通过 `for` 循环遍历字符串中的每一个字符,判断该字符是否为数字字符,如果是则将 `count` 加一。最后输出统计的数字字符个数即可。
相关推荐
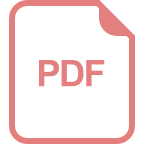
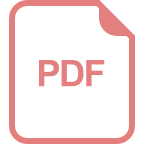
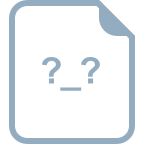












