python实现gru-attention时间序列预测
时间: 2023-08-26 07:04:50 浏览: 87
GRU-Attention是一种用于时间序列预测的深度学习模型。下面我来简单介绍一下GRU-Attention的实现过程。
首先,我们需要导入必要的库:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from keras.models import Sequential, Model
from keras.layers import Dense, GRU, Input, RepeatVector, TimeDistributed, Lambda, Activation, Dot, Concatenate
from keras.optimizers import Adam
from keras.callbacks import EarlyStopping
```
接着,我们需要准备数据集。这里以一个简单的sin函数为例:
```python
x = np.arange(0, 100, 0.1)
y = np.sin(x)
```
由于GRU-Attention是一种序列模型,我们需要将数据集转化为时间序列数据。这里我们取时间窗口为10,即每个时间点预测后面10个时间点的数值:
```python
window_size = 10
X = []
Y = []
for i in range(len(y) - window_size):
X.append(y[i:i+window_size])
Y.append(y[i+window_size:i+window_size+1])
X = np.array(X)
Y = np.array(Y)
```
接着,我们需要定义GRU-Attention模型。这里我们使用两层GRU和一个Attention层:
```python
def attention(inputs):
# inputs.shape = (batch_size, time_steps, input_dim)
input_dim = int(inputs.shape[2])
a = Permute((2, 1))(inputs)
a = Dense(time_steps, activation='softmax')(a)
a = Lambda(lambda x: K.mean(x, axis=1), name='dim_reduction')(a)
a = RepeatVector(input_dim)(a)
a_probs = Permute((2, 1), name='attention_vec')(a)
output_attention_mul = Multiply(name='attention_mul')([inputs, a_probs])
return output_attention_mul
model = Sequential()
model.add(GRU(64, input_shape=(window_size, 1), return_sequences=True))
model.add(GRU(32, return_sequences=True))
model.add(Attention())
model.add(TimeDistributed(Dense(1)))
model.add(Activation('linear'))
model.compile(loss='mean_squared_error', optimizer=Adam(lr=0.001))
```
接着,我们可以开始训练模型:
```python
early_stopping = EarlyStopping(monitor='val_loss', patience=10)
history = model.fit(X_train, Y_train, validation_split=0.2, epochs=100, batch_size=64, callbacks=[early_stopping])
```
最后,我们可以用模型进行预测:
```python
y_pred = model.predict(X)
plt.plot(y, label='true')
plt.plot(y_pred[:, -1, 0], label='predicted')
plt.legend()
plt.show()
```
以上就是GRU-Attention时间序列预测的实现过程。当然,具体的模型参数和数据集处理方式需要根据实际情况进行调整。
相关推荐
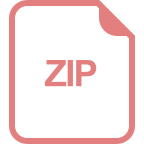
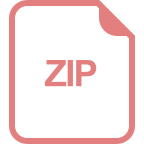
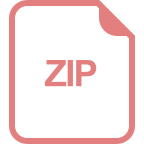














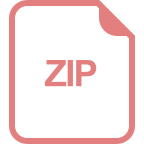