torch.nonzero(max_ious >= iou_threshold)
时间: 2024-03-03 14:50:35 浏览: 126
`torch.nonzero()` 函数是 PyTorch 中用来找到张量中非零元素的下标的函数。具体来说,对于一个输入张量,它将返回一个二维张量,该张量的每一行都是一个非零元素的下标。下面是一个示例代码:
```
import torch
x = torch.tensor([[0, 1, 0],
[2, 0, 3],
[0, 4, 0]])
nonzero_idx = torch.nonzero(x)
print(nonzero_idx)
```
输出结果为:
```
tensor([[0, 1],
[1, 0],
[1, 2],
[2, 1]])
```
在这个示例中,我们首先创建了一个形状为(3, 3)的张量,然后使用`torch.nonzero()`函数找到了其中所有的非零元素的下标,并将其打印出来。
在你的代码中,`max_ious >= iou_threshold` 是一个布尔型张量,表示哪些 anchor 与任意一个 ground-truth box 的 IoU 值大于等于阈值。然后,`torch.nonzero()`函数将找到这个张量中所有为 True 的元素的下标,并返回一个二维张量,该张量的每一行都是一个满足条件的元素的下标。
相关问题
class SupConLossV2(nn.Module): def __init__(self, temperature=0.2, iou_threshold=0.5): super().__init__() self.temperature = temperature self.iou_threshold = iou_threshold def forward(self, features, labels, ious): if len(labels.shape) == 1: labels = labels.reshape(-1, 1) # mask of shape [None, None], mask_{i, j}=1 if sample i and sample j have the same label label_mask = torch.eq(labels, labels.T).float().cuda() similarity = torch.div( torch.matmul(features, features.T), self.temperature) # for numerical stability sim_row_max, _ = torch.max(similarity, dim=1, keepdim=True) similarity = similarity - sim_row_max.detach() # mask out self-contrastive logits_mask = torch.ones_like(similarity) logits_mask.fill_diagonal_(0) exp_sim = torch.exp(similarity) mask = logits_mask * label_mask keep = (mask.sum(1) != 0 ) & (ious >= self.iou_threshold) log_prob = torch.log( (exp_sim[keep] * mask[keep]).sum(1) / (exp_sim[keep] * logits_mask[keep]).sum(1) ) loss = -log_prob return loss.mean()
这是一个实现对比学习(contrastive learning)损失函数的 PyTorch 模块。对比学习是一种无监督学习方法,它通过最大化相似样本的相似度,最小化不相似样本的相似度来学习特征表示。该模块的输入是特征张量、标签张量和 IOU 张量,输出是对比学习损失。在 forward 方法中,首先计算了相似度矩阵,即特征张量的内积矩阵除以温度参数,同时使用标签张量生成了掩码矩阵,其中掩码矩阵的元素值为 1 表示对应样本的标签相同,元素值为 0 表示对应样本的标签不同。然后对相似度矩阵进行了行归一化,并通过掩码矩阵和 IOU 张量筛选出需要进行对比学习的样本对,最后计算了对数概率损失并返回平均损失。该损失函数的目标是最小化相似样本之间的欧几里得距离,最大化不相似样本之间的欧几里得距离。
修改# 对每个相机图像进行目标检测 detections1 = model(image1) # 检测结果1 detections2 = model(image2) # 检测结果2 detections3 = model(image3) # 检测结果3 # 对检测结果进行筛选 filtered_detections1 = non_max_suppression(detections1, confidence_threshold, iou_threshold) filtered_detections2 = non_max_suppression(detections2, confidence_threshold, iou_threshold) filtered_detections3 = non_max_suppression(detections3, confidence_threshold, iou_threshold)报错TypeError: conv2d(): argument 'input' (position 1) must be Tensor, not numpy.ndarray
这个错误是因为YOLOv7输出的是一个numpy数组,而非PyTorch张量,因此无法在PyTorch中进一步处理。您需要将numpy数组转换为PyTorch张量,可以使用以下代码:
```
import torch
import numpy as np
# 转换图像为PyTorch张量
image1 = torch.from_numpy(np.ascontiguousarray(image1)).to(device)
image2 = torch.from_numpy(np.ascontiguousarray(image2)).to(device)
image3 = torch.from_numpy(np.ascontiguousarray(image3)).to(device)
# 检测目标
detections1 = model(image1)
detections2 = model(image2)
detections3 = model(image3)
# 转换检测结果为PyTorch张量
detections1 = [torch.from_numpy(x) for x in detections1]
detections2 = [torch.from_numpy(x) for x in detections2]
detections3 = [torch.from_numpy(x) for x in detections3]
# 对检测结果进行筛选
filtered_detections1 = non_max_suppression(detections1, confidence_threshold, iou_threshold)
filtered_detections2 = non_max_suppression(detections2, confidence_threshold, iou_threshold)
filtered_detections3 = non_max_suppression(detections3, confidence_threshold, iou_threshold)
```
这将确保您的检测结果可以在PyTorch中进一步处理。
阅读全文
相关推荐
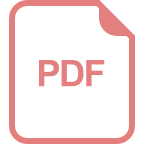
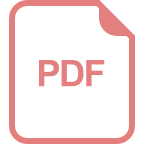

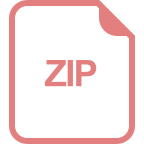
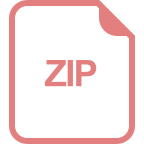

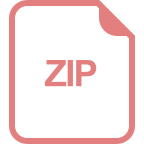
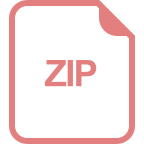
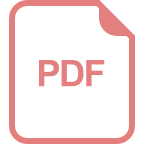
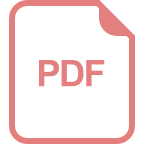
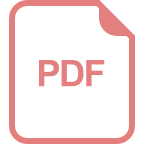
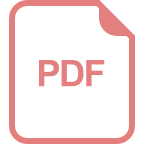
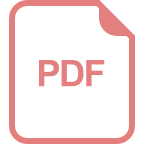
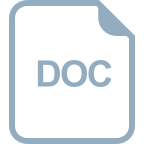
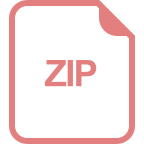