用Keil写一个8路流水灯,两侧各一个LED同时亮起,之后依次向中间聚拢,然后在展开,接着,在8个灯闪三次,为一次循环...
时间: 2024-03-01 15:49:45 浏览: 13
好的,这是一个比较常见的MCU实践题目,我可以给你提供基本的思路和代码片段。假设使用的是STM32F1系列的开发板,以下是大致的实现流程:
1. 首先定义8个LED的端口和引脚号,如下所示:
```c
#define LED0_PORT GPIOB
#define LED0_PIN GPIO_Pin_0
#define LED1_PORT GPIOB
#define LED1_PIN GPIO_Pin_1
#define LED2_PORT GPIOB
#define LED2_PIN GPIO_Pin_2
#define LED3_PORT GPIOB
#define LED3_PIN GPIO_Pin_3
#define LED4_PORT GPIOB
#define LED4_PIN GPIO_Pin_4
#define LED5_PORT GPIOB
#define LED5_PIN GPIO_Pin_5
#define LED6_PORT GPIOB
#define LED6_PIN GPIO_Pin_6
#define LED7_PORT GPIOB
#define LED7_PIN GPIO_Pin_7
```
2. 在main函数中初始化GPIO
```c
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); // 使能 GPIOB 时钟
GPIO_InitStructure.GPIO_Pin = LED0_PIN | LED1_PIN | LED2_PIN | LED3_PIN | LED4_PIN | LED5_PIN | LED6_PIN | LED7_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
```
3. 在main函数中定义一个数组来存储LED的状态,使用循环语句控制LED的流水效果:
```c
uint16_t led_array[8] = {LED0_PIN, LED1_PIN, LED2_PIN, LED3_PIN, LED4_PIN, LED5_PIN, LED6_PIN, LED7_PIN};
while (1) {
int i, j;
for (i = 0, j = 7; i < 4; i++, j--) {
GPIO_SetBits(LED0_PORT, led_array[i]);
GPIO_SetBits(LED7_PORT, led_array[j]);
delay(200);
GPIO_ResetBits(LED0_PORT, led_array[i]);
GPIO_ResetBits(LED7_PORT, led_array[j]);
}
for (i = 1, j = 6; i < 3; i++, j--) {
GPIO_SetBits(LED1_PORT, led_array[i]);
GPIO_SetBits(LED6_PORT, led_array[j]);
delay(200);
GPIO_ResetBits(LED1_PORT, led_array[i]);
GPIO_ResetBits(LED6_PORT, led_array[j]);
}
for (i = 2, j = 5; i < 2; i++, j--) {
GPIO_SetBits(LED2_PORT, led_array[i]);
GPIO_SetBits(LED5_PORT, led_array[j]);
delay(200);
GPIO_ResetBits(LED2_PORT, led_array[i]);
GPIO_ResetBits(LED5_PORT, led_array[j]);
}
for (i = 3, j = 4; i < 1; i++, j--) {
GPIO_SetBits(LED3_PORT, led_array[i]);
GPIO_SetBits(LED4_PORT, led_array[j]);
delay(200);
GPIO_ResetBits(LED3_PORT, led_array[i]);
GPIO_ResetBits(LED4_PORT, led_array[j]);
}
// 8个LED闪三次
for (i = 0; i < 8; i++) {
int k;
for (k = 0; k < 3; k++) {
GPIO_SetBits(LED0_PORT, led_array[i]);
delay(100);
GPIO_ResetBits(LED0_PORT, led_array[i]);
delay(100);
}
}
}
```
4. 最后在程序中定义一个延时函数:
```c
void delay(uint32_t nCount) {
for(; nCount != 0; nCount--);
}
```
这样就完成了一个简单的8路流水灯,两侧各一个LED同时亮起,之后依次向中间聚拢,然后在展开,接着,在8个灯闪三次,为一次循环的程序。
相关推荐













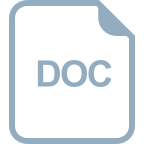
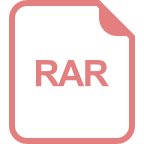