使用sklearn库的两种算法进行房价预测分析
时间: 2023-07-25 22:32:40 浏览: 88
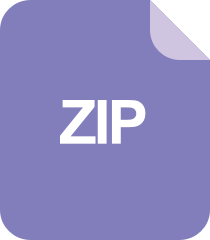
基于Flask + mysql + scikit-learn + 多元线性回归算法+echarts可视化实现的房价预测项目源码
下面分别介绍使用sklearn库中线性回归算法和随机森林算法进行房价预测分析的步骤:
1. 线性回归算法:
(1)导入必要的库和数据集
```
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import pandas as pd
data = pd.read_csv('house_price.csv')
```
(2)数据预处理
```
X = data.drop(['Price'], axis=1)
y = data['Price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
```
(3)训练模型并预测
```
model = LinearRegression()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
```
(4)评估模型性能
```
mse = mean_squared_error(y_test, y_pred)
print("Mean squared error: ", mse)
```
2. 随机森林算法:
(1)导入必要的库和数据集
```
from sklearn.ensemble import RandomForestRegressor
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import pandas as pd
data = pd.read_csv('house_price.csv')
```
(2)数据预处理
```
X = data.drop(['Price'], axis=1)
y = data['Price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
```
(3)训练模型并预测
```
model = RandomForestRegressor(n_estimators=100, random_state=0)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
```
(4)评估模型性能
```
mse = mean_squared_error(y_test, y_pred)
print("Mean squared error: ", mse)
```
需要注意的是,在使用随机森林算法进行房价预测时,需要设置n_estimators参数,该参数表示集成中树的数量。在实际应用中,需要通过交叉验证等方法来选择最优的n_estimators值。
阅读全文
相关推荐
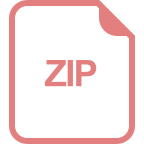

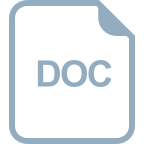
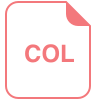
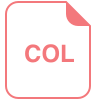
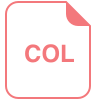










