用Python写一段通过GPU解码RTSP视频流的代码
时间: 2023-03-11 17:16:27 浏览: 288
可以使用FFmpeg库中的avcodec_decode_video2函数来解码RTSP视频流,下面是一段示例代码:import ffmpegin_stream = ffmpeg.input('rtsp://example.com/stream.sdp')
out_codec = ffmpeg.output(in_stream, 'decoded_video.mp4', vcodec= 'h264_cuvid', cuda=True)ffmpeg.run(out_codec)
相关问题
用python写一段代码,要求能实现通过cv2播放rtsp视频流
在Python中,你可以使用OpenCV (cv2) 库来读取并播放RTSP视频流。以下是一个简单的例子:
```python
import cv2
# 创建 VideoCapture 对象,传入 RTSP 地址
cap = cv2.VideoCapture('rtsp://your_rts_stream_url')
# 检查是否成功打开
if not cap.isOpened():
print("Error opening video stream or file")
else:
# 设置循环,持续播放视频
while True:
# 读取下一帧
ret, frame = cap.read()
# 如果读取失败,跳出循环
if not ret:
break
# 显示视频帧
cv2.imshow('RTSP Video', frame)
# 等待按键,按 'q' 键退出
key = cv2.waitKey(1)
if key == ord('q'):
break
# 关闭窗口和释放资源
cap.release()
cv2.destroyAllWindows()
帮我用python写一段优化rtsp取流延迟的代码
在Python中,我们可以使用`cv2.VideoCapture`结合`threading`模块来优化RTSP流的处理,减少延迟。这里是一个基本示例,展示如何在单独的线程中抓取帧并存储,主程序则专注于处理这些帧:
```python
import cv2
import threading
class FrameGrabber(threading.Thread):
def __init__(self, rtsp_url, queue):
threading.Thread.__init__(self)
self.cap = cv2.VideoCapture(rtsp_url)
self.queue = queue
self.daemon = True
def run(self):
while True:
ret, frame = self.cap.read()
if ret:
# 将帧添加到队列中
self.queue.put(frame)
else:
break
def process_frames(queue):
while True:
frame = queue.get() # 从队列中取出帧
# 这里是对帧进行处理的代码,例如图像识别等
processed_frame = process_image(frame)
# 处理后的帧可以进一步保存、显示或者传输
# ...
# 创建一个帧队列
frame_queue = queue.Queue()
# 创建并启动帧获取线程
grabber_thread = FrameGrabber(rtsp_url="your_rtsp_stream", queue=frame_queue)
grabber_thread.start()
# 启动帧处理线程
processor_thread = threading.Thread(target=process_frames, args=(frame_queue,))
processor_thread.start()
# 等待所有线程结束
grabber_thread.join()
processor_thread.join()
```
在这个例子中,`FrameGrabber`线程负责获取帧并将它们放入队列,而`process_frames`函数从队列中取出帧并进行后续处理。这样就实现了RTSP流的高效抓取和延迟优化。
注意替换`rtsp_url="your_rtsp_stream"`为实际的RTSP流地址,并根据需求调整`process_image()`函数的具体内容。
阅读全文
相关推荐
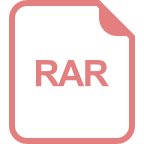
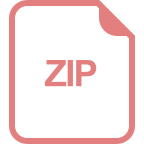
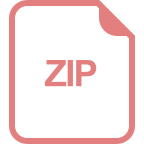













