使用Java实现快速排序的几种方法和代码?
时间: 2023-08-31 18:27:15 浏览: 111
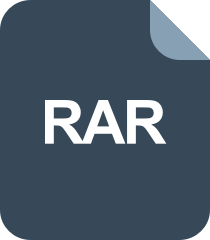
快速排序优化的几种方法代码实现
### 回答1:
快速排序是一种常用的排序算法,使用 Java 实现快速排序有以下几种方法:
1. 递归方法
```
public class QuickSort {
public static void sort(int[] arr, int low, int high) {
if (arr == null || arr.length == 0)
return;
if (low >= high)
return;
int middle = low + (high - low) / 2;
int pivot = arr[middle];
int i = low, j = high;
while (i <= j) {
while (arr[i] < pivot) {
i++;
}
while (arr[j] > pivot) {
j--;
}
if (i <= j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
i++;
j--;
}
}
if (low < j)
sort(arr, low, j);
if (high > i)
sort(arr, i, high);
}
public static void main(String[] args) {
int[] arr = {9, 8, 7, 6, 5, 4, 3, 2, 1};
sort(arr, 0, arr.length-1);
for (int i : arr) {
System.out.print(i + " ");
}
}
}
```
2. 非递归方法
```
import java.util.Stack;
public class QuickSort2 {
public static void sort(int[] arr) {
if (arr == null || arr.length == 0)
return;
Stack<Integer> stack = new Stack<Integer>();
stack.push(0);
stack.push(arr.length - 1);
while (!stack.isEmpty()) {
int high = stack.pop();
int low = stack.pop();
int pivot = partition(arr, low, high);
if (pivot - 1 > low) {
stack.push(low);
stack.push(pivot - 1);
}
if (pivot + 1 < high) {
stack.push(pivot + 1);
stack.push(high);
}
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
int i = low + 1;
int j = high;
while (i <= j) {
while (i <= j && arr[i] < pivot) {
i++;
}
while (i <= j && arr[j] > pivot) {
j--;
}
if (i <= j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j]
### 回答2:
快速排序是一种常用的排序算法,它的基本思想是通过划分将数据分成较小和较大的两部分,递归地对两部分进行排序,最终实现整个序列的有序。
在Java中实现快速排序的几种常用方法如下:
1. 递归实现:
```java
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int partitionIndex = partition(arr, low, high);
quickSort(arr, low, partitionIndex - 1);
quickSort(arr, partitionIndex + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
```
2. 非递归实现:
```java
public static void quickSort(int[] arr) {
if (arr == null || arr.length <= 1) {
return;
}
Stack<Integer> stack = new Stack<>();
stack.push(0);
stack.push(arr.length - 1);
while (!stack.isEmpty()) {
int high = stack.pop();
int low = stack.pop();
int partitionIndex = partition(arr, low, high);
if (low < partitionIndex - 1) {
stack.push(low);
stack.push(partitionIndex - 1);
}
if (high > partitionIndex + 1) {
stack.push(partitionIndex + 1);
stack.push(high);
}
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
```
以上就是使用Java实现快速排序的几种常用方法和代码。快速排序的时间复杂度为O(nlogn),是一种高效的排序算法。
### 回答3:
快速排序是一种经典的排序算法,可以使用多种方式实现。下面介绍两种常见的 Java 实现方法。
一种常见的快速排序实现方法是使用递归。首先选择一个基准元素(通常是数组的第一个元素),将数组分成两个部分,小于基准元素的放在左边,大于基准元素的放在右边。然后再递归地对左右两个部分进行快速排序。
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivot = partition(arr, low, high);
quickSort(arr, low, pivot - 1);
quickSort(arr, pivot + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
}
```
另一种常见的快速排序实现方法是使用栈。首先将初始的 low 和 high 压入栈中,然后循环处理栈顶的元素,直到栈为空。在每次循环中,先弹出栈顶的 low 和 high,并执行划分过程,将得到的两个新的 low 和 high 压入栈中。
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
Stack<Integer> stack = new Stack<>();
stack.push(low);
stack.push(high);
while (!stack.isEmpty()) {
high = stack.pop();
low = stack.pop();
int pivot = partition(arr, low, high);
if (low < pivot - 1) {
stack.push(low);
stack.push(pivot - 1);
}
if (pivot + 1 < high) {
stack.push(pivot + 1);
stack.push(high);
}
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
}
```
这两种实现方法都基于相同的快速排序思想,只是在具体的实现上略有不同。快速排序的时间复杂度通常为 O(nlogn),是一种效率较高的排序算法。
阅读全文
相关推荐
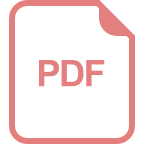
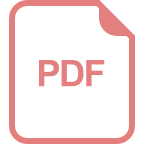
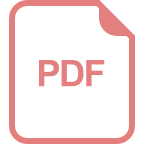
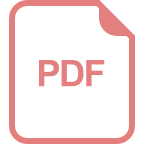
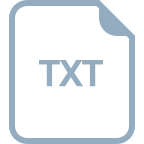
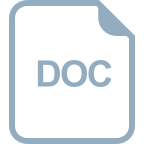
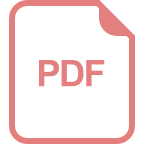
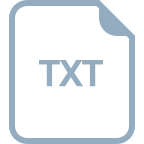
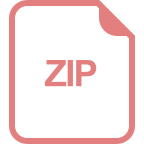
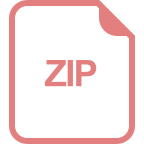
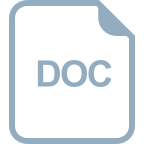
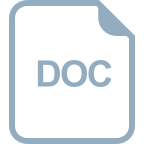
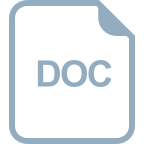
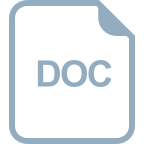
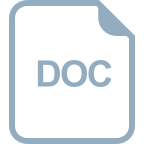