# Split the dataset x_train, x_test, y_train, y_test = train_test_split(x_train, y_train, test_size=0.2, random_state=42) x_train, x_validate, y_train, y_validate = train_test_split(x_train, y_train, test_size=0.1, random_state=999) # Resize images for i in range(len(x_train)): img = Image.fromarray(x_train[i]) img = img.resize((224, 224)) x_train[i] = np.array(img) for i in range(len(x_validate)): img = Image.fromarray(x_validate[i]) img = img.resize((224, 224)) x_validate[i] = np.array(img) # Reshape images x_train = x_train.reshape(x_train.shape[0], 224, 224, 3) x_validate = x_validate.reshape(x_validate.shape[0], 224, 224, 3)代码段报错TypeError: Cannot handle this data type如何解决
时间: 2023-12-24 15:25:47 浏览: 27
这个错误提示表明你试图使用无法处理的数据类型。可能原因是你的代码中存在错误,或者是你的输入数据类型不正确。建议你检查代码中的变量和数组的数据类型,确保它们符合你的预期。你可以使用print语句来打印变量的数据类型和值,以便更好地了解代码的执行过程。另外,你可以尝试将输入数据类型转换为正确的类型,例如使用numpy中的astype方法将数据类型转换为float或int类型。
相关问题
dataset数据集分为x_train,y_train,x_test,y_testatlab
在 Matlab 中,可以使用 `matfile` 函数来读取和处理数据集。一般来说,将数据集分为训练数据和测试数据的过程可以如下进行:
1. 读取整个数据集文件,可以使用 `matfile` 函数读取数据集的 `.mat` 文件,例如:
```matlab
data = matfile('dataset.mat');
```
2. 随机打乱数据集,可以使用 `randperm` 函数生成随机索引,将数据集打乱,例如:
```matlab
idx = randperm(size(data.images, 4));
shuffled_images = data.images(:, :, :, idx);
shuffled_labels = data.labels(idx);
```
3. 将数据集分为训练数据和测试数据,可以使用 `floor` 函数计算分割点的索引,例如:
```matlab
n = size(shuffled_images, 4);
split = floor(n * 0.7);
x_train = shuffled_images(:, :, :, 1:split);
y_train = shuffled_labels(1:split);
x_test = shuffled_images(:, :, :, split+1:end);
y_test = shuffled_labels(split+1:end);
```
其中 `0.7` 是训练数据所占的比例,可以根据实际情况进行调整。分割后的 `x_train`、`y_train`、`x_test` 和 `y_test` 可以用来训练和测试模型。注意,在这个例子中,数据集是由四维数组构成的,因此需要在索引的时候指定维度。
x_train, x_test, y_train, y_test = train_test_split(x_Dataset, y_Dataset, test_size=0.2,random_state=42)
这是一个用于数据集划分的代码,其中 x_Dataset 和 y_Dataset 是输入的数据集,train_test_split 函数将其随机划分为训练集和测试集,其中测试集占总数据集的 20%,random_state=42 表示使用固定的随机种子以确保每次划分的结果一致。
相关推荐
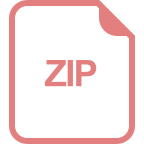
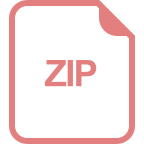












