python使用Opencv的circle函数绘制圆转成使用polylines函数绘制圆,并将外轮廓坐标点输出
时间: 2024-03-19 22:44:47 浏览: 21
好的,这是一个关于使用OpenCV在Python中绘制圆的问题。你可以使用`cv2.polylines()`函数来绘制圆。具体步骤如下:
1. 导入必要的库并读取图像。
```python
import cv2
import numpy as np
# Read image
img = np.zeros((200, 200, 3), dtype=np.uint8)
```
2. 定义一个圆的半径和圆心坐标。
```python
radius = 50
center = (100, 100)
```
3. 定义一个空列表来存储圆的所有点。
```python
points = []
```
4. 遍历圆的每个角度,使用三角函数计算出每个点的坐标。
```python
for angle in range(0, 360, 10):
x = int(center[0] + radius * np.cos(angle * np.pi / 180))
y = int(center[1] + radius * np.sin(angle * np.pi / 180))
points.append((x, y))
```
5. 最后,使用`cv2.polylines()`函数绘制圆,并将所有坐标点输出。
```python
contours = [np.array(points)]
cv2.polylines(img, contours, True, (255, 0, 0), 2)
print("Contours:", contours[0])
```
这里的`img`是你要绘制圆的图像,`True`表示绘制封闭的轮廓,`(255, 0, 0)`表示轮廓的颜色,`2`表示轮廓的线宽。
输出的`contours[0]`是一个numpy数组,包含了所有圆的坐标点。
完整代码如下:
```python
import cv2
import numpy as np
# Read image
img = np.zeros((200, 200, 3), dtype=np.uint8)
# Define circle center and radius
radius = 50
center = (100, 100)
# Define a list to store all the points on the circle
points = []
# Calculate the coordinates of each point on the circle
for angle in range(0, 360, 10):
x = int(center[0] + radius * np.cos(angle * np.pi / 180))
y = int(center[1] + radius * np.sin(angle * np.pi / 180))
points.append((x, y))
# Draw the circle using polylines function
contours = [np.array(points)]
cv2.polylines(img, contours, True, (255, 0, 0), 2)
# Print out all the contour points
print("Contours:", contours[0])
cv2.imshow("Circle", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
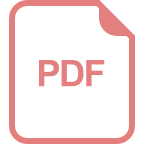
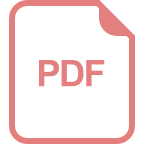
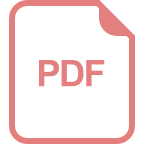














