请写出stc32g12k128单片机的usb配置程序
时间: 2023-10-10 09:10:13 浏览: 276
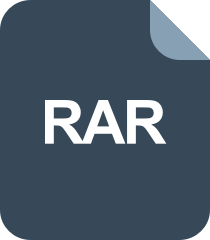
单片机的USB驱动程序

STC32G12K128 支持 USB 设备模式,可以通过 USB 接口与计算机进行通信。以下是 STC32G12K128 的 USB 配置程序示例:
```
#include <stc12.h>
#define USB_DEVICE_DESC_SIZE 18
#define USB_CONFIG_DESC_SIZE 9
#define USB_INTERFACE_DESC_SIZE 9
#define USB_ENDPOINT_DESC_SIZE 7
__code unsigned char DeviceDescriptor[USB_DEVICE_DESC_SIZE] = {
0x12, // bLength
0x01, // bDescriptorType
0x00, 0x02, // bcdUSB
0x02, // bDeviceClass
0x00, // bDeviceSubClass
0x00, // bDeviceProtocol
0x40, // bMaxPacketSize0
0x1C, 0x4D, // idVendor
0x55, 0x00, // idProduct
0x00, 0x01, // bcdDevice
0x01, // iManufacturer
0x02, // iProduct
0x03, // iSerialNumber
0x01 // bNumConfigurations
};
__code unsigned char ConfigDescriptor[USB_CONFIG_DESC_SIZE] = {
0x09, // bLength
0x02, // bDescriptorType
0x22, 0x00, // wTotalLength
0x01, // bNumInterfaces
0x01, // bConfigurationValue
0x00, // iConfiguration
0xC0, // bmAttributes
0x32 // bMaxPower
};
__code unsigned char InterfaceDescriptor[USB_INTERFACE_DESC_SIZE] = {
0x09, // bLength
0x04, // bDescriptorType
0x00, // bInterfaceNumber
0x00, // bAlternateSetting
0x02, // bNumEndpoints
0xFF, // bInterfaceClass
0x00, // bInterfaceSubClass
0x00, // bInterfaceProtocol
0x00 // iInterface
};
__code unsigned char EndpointDescriptor[USB_ENDPOINT_DESC_SIZE] = {
0x07, // bLength
0x05, // bDescriptorType
0x81, // bEndpointAddress
0x02, // bmAttributes
0x40, 0x00, // wMaxPacketSize
0x01 // bInterval
};
__code unsigned char StringDescriptor0[4] = {
0x04, // bLength
0x03, // bDescriptorType
0x09, 0x04 // wLANGID
};
__code unsigned char StringDescriptor1[16] = {
0x10, // bLength
0x03, // bDescriptorType
'S', 0x00, // manufacturer
'T', 0x00,
'C', 0x00,
' ', 0x00,
'M', 0x00, // product
'C', 0x00,
'U', 0x00
};
__code unsigned char StringDescriptor2[16] = {
0x10, // bLength
0x03, // bDescriptorType
'S', 0x00, // manufacturer
'T', 0x00,
'C', 0x00,
' ', 0x00,
'U', 0x00, // product
'S', 0x00,
'B', 0x00
};
__code unsigned char StringDescriptor3[16] = {
0x10, // bLength
0x03, // bDescriptorType
'1', 0x00, // serial number
'2', 0x00,
'3', 0x00,
'4', 0x00,
'5', 0x00,
'6', 0x00
};
void initUSB() {
USBCS = 0x00; // 清空 USB 中断标志
USBCS |= 0x04; // 允许 USB 中断
USBCS |= 0x02; // 允许 USB 模块
}
void main() {
initUSB();
while (1) {
// 待机状态
}
}
// USB 中断服务程序
void USB_ISR() interrupt 17 {
if (USBCS & 0x01) {
// USB RESET 中断
USBCS &= ~0x01;
} else if (USBCS & 0x02) {
// USB SOF 中断
USBCS &= ~0x02;
} else if (USBCS & 0x08) {
// USB SETUP 中断
USBCS &= ~0x08;
// 处理 SETUP 数据包
} else if (USBCS & 0x10) {
// USB IN 中断
USBCS &= ~0x10;
// 发送 IN 数据包
} else if (USBCS & 0x20) {
// USB OUT 中断
USBCS &= ~0x20;
// 接收 OUT 数据包
}
}
```
在上述程序中,我们首先定义了一些 USB 描述符,包括设备描述符、配置描述符、接口描述符、端点描述符和字符串描述符。然后在 `initUSB()` 函数中,我们配置了 USB 中断使能和 USB 模块使能。在 `main()` 函数中,我们进入了待机状态,等待 USB 中断的触发。最后,我们定义了一个 `USB_ISR()` 中断服务程序,用于处理 USB RESET、USB SOF、USB SETUP、USB IN 和 USB OUT 等中断事件。
需要注意的是,STC32G12K128 的 USB 功能比较复杂,需要开发者仔细查阅数据手册和技术文档,以确保正确地配置和使用 USB 功能。同时,在进行 USB 开发时,还需要注意 USB 时序、USB 协议和 USB 设备驱动等方面的问题。
阅读全文
相关推荐
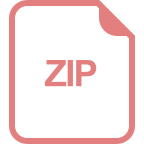



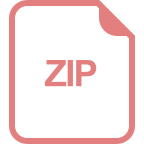
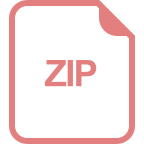
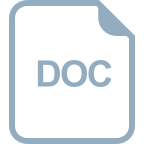
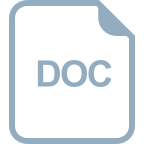
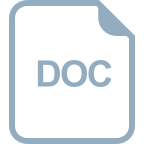







