html +css 实现一个表头固定表体自动循环无缝滚动的表格,鼠标悬浮时停止,滚动出去的数据自动插入后边
时间: 2023-12-03 09:42:36 浏览: 479
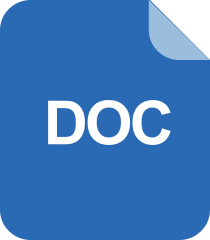
自动滚屏(HTML经典用例)

实现表头固定表体自动循环无缝滚动的表格,可以使用以下步骤:
1. HTML 结构
首先,我们需要先搭建表格的 HTML 结构。代码如下:
```html
<div class="table-wrap">
<table>
<thead>
<tr>
<th>表头1</th>
<th>表头2</th>
<th>表头3</th>
<th>表头4</th>
<th>表头5</th>
</tr>
</thead>
<tbody>
<tr>
<td>数据1-1</td>
<td>数据1-2</td>
<td>数据1-3</td>
<td>数据1-4</td>
<td>数据1-5</td>
</tr>
<tr>
<td>数据2-1</td>
<td>数据2-2</td>
<td>数据2-3</td>
<td>数据2-4</td>
<td>数据2-5</td>
</tr>
<!-- 其他数据行 -->
</tbody>
</table>
</div>
```
2. CSS 样式
接下来,我们需要设置表格的 CSS 样式,包括表格的宽度、高度、边框等属性,以及表头的固定和表体的滚动效果。代码如下:
```css
.table-wrap {
width: 100%;
height: 300px;
overflow: hidden;
}
table {
width: 100%;
border-collapse: collapse;
}
th,
td {
border: 1px solid #ccc;
padding: 8px;
text-align: center;
}
thead {
position: sticky;
top: 0;
background-color: #fff;
}
tbody {
animation: scroll 20s linear infinite;
}
@keyframes scroll {
0% {
transform: translateY(0);
}
100% {
transform: translateY(-100%);
}
}
```
其中,`.table-wrap` 是表格容器的类名,设置了容器的宽度、高度和溢出隐藏;`table` 是表格的标签选择器,设置了表格的宽度和边框合并;`th` 和 `td` 分别是表头和数据行的样式,设置了单元格的边框、内边距和居中对齐;`thead` 是表头的选择器,设置了表头的固定和背景颜色;`tbody` 是表体的选择器,设置了表体的滚动效果和动画时间。
3. JavaScript 交互
最后,我们需要使用 JavaScript 实现鼠标悬浮停止滚动和滚动出去的数据自动插入的效果。代码如下:
```javascript
const tableWrap = document.querySelector('.table-wrap')
let timer = null
tableWrap.addEventListener('mouseover', () => {
clearInterval(timer)
})
tableWrap.addEventListener('mouseout', () => {
timer = setInterval(() => {
const firstRow = tableWrap.querySelector('tbody tr:first-child')
const cloneRow = firstRow.cloneNode(true)
tableWrap.querySelector('tbody').appendChild(cloneRow)
firstRow.remove()
}, 2000)
})
timer = setInterval(() => {
const firstRow = tableWrap.querySelector('tbody tr:first-child')
const cloneRow = firstRow.cloneNode(true)
tableWrap.querySelector('tbody').appendChild(cloneRow)
firstRow.remove()
}, 2000)
```
其中,`tableWrap` 是表格容器的 DOM 元素,使用 `querySelector` 方法获取;`timer` 是定时器变量;`mouseover` 和 `mouseout` 是鼠标悬浮和移出容器的事件,分别清除和启动定时器;定时器中的代码实现了滚动出去的数据自动插入后边的效果,具体实现方法是先使用 `querySelector` 获取表格数据的第一行元素 `firstRow`,然后使用 `cloneNode` 方法克隆这一行元素,再使用 `appendChild` 方法将克隆的行元素添加到表格数据的最后一行,最后使用 `remove` 方法删除原来的第一行元素。
综上所述,HTML、CSS 和 JavaScript 的代码实现如下:
```html
<div class="table-wrap">
<table>
<thead>
<tr>
<th>表头1</th>
<th>表头2</th>
<th>表头3</th>
<th>表头4</th>
<th>表头5</th>
</tr>
</thead>
<tbody>
<tr>
<td>数据1-1</td>
<td>数据1-2</td>
<td>数据1-3</td>
<td>数据1-4</td>
<td>数据1-5</td>
</tr>
<tr>
<td>数据2-1</td>
<td>数据2-2</td>
<td>数据2-3</td>
<td>数据2-4</td>
<td>数据2-5</td>
</tr>
<!-- 其他数据行 -->
</tbody>
</table>
</div>
<style>
.table-wrap {
width: 100%;
height: 300px;
overflow: hidden;
}
table {
width: 100%;
border-collapse: collapse;
}
th,
td {
border: 1px solid #ccc;
padding: 8px;
text-align: center;
}
thead {
position: sticky;
top: 0;
background-color: #fff;
}
tbody {
animation: scroll 20s linear infinite;
}
@keyframes scroll {
0% {
transform: translateY(0);
}
100% {
transform: translateY(-100%);
}
}
</style>
<script>
const tableWrap = document.querySelector('.table-wrap')
let timer = null
tableWrap.addEventListener('mouseover', () => {
clearInterval(timer)
})
tableWrap.addEventListener('mouseout', () => {
timer = setInterval(() => {
const firstRow = tableWrap.querySelector('tbody tr:first-child')
const cloneRow = firstRow.cloneNode(true)
tableWrap.querySelector('tbody').appendChild(cloneRow)
firstRow.remove()
}, 2000)
})
timer = setInterval(() => {
const firstRow = tableWrap.querySelector('tbody tr:first-child')
const cloneRow = firstRow.cloneNode(true)
tableWrap.querySelector('tbody').appendChild(cloneRow)
firstRow.remove()
}, 2000)
</script>
```
阅读全文
相关推荐
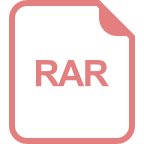
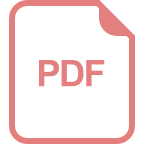
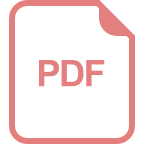
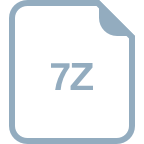
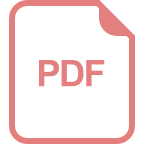
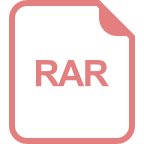
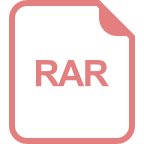
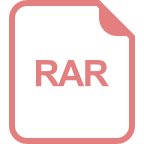
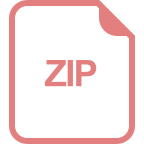
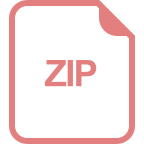
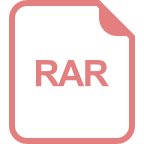