springboot怎么下载文件
时间: 2023-09-03 22:15:31 浏览: 50
在Spring Boot中下载文件有多种方式,以下是其中两种:
1. 使用ResponseEntity<byte[]>返回文件
```java
@GetMapping("/downloadFile")
public ResponseEntity<byte[]> downloadFile() throws IOException {
// 读取文件
File file = new File("path/to/file.txt");
byte[] data = FileUtils.readFileToByteArray(file);
// 设置响应头
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", file.getName());
headers.setContentLength(data.length);
// 返回ResponseEntity
return new ResponseEntity<>(data, headers, HttpStatus.OK);
}
```
2. 使用OutputStream写入文件
```java
@GetMapping("/downloadFile")
public void downloadFile(HttpServletResponse response) throws IOException {
// 读取文件
File file = new File("path/to/file.txt");
byte[] data = FileUtils.readFileToByteArray(file);
// 设置响应头
response.setContentType(MediaType.APPLICATION_OCTET_STREAM_VALUE);
response.setHeader("Content-Disposition", "attachment; filename=" + file.getName());
response.setContentLength(data.length);
// 写入输出流
OutputStream outputStream = response.getOutputStream();
outputStream.write(data);
outputStream.flush();
outputStream.close();
}
```
以上两种方式都可以实现文件下载,第一种方式使用了ResponseEntity来封装响应,第二种方式直接使用HttpServletResponse写入响应。根据实际情况选择合适的方式即可。
相关推荐
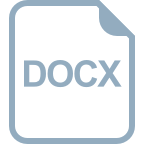















