采用三级队列调度策略,其中一级队列采用非抢占式短作业优先,二级队列采用轮转时间片,三级队列采用FCFS,进程的到达时间和运行时间随机生成,按照时间顺序执行进程,优先级抢占,低队列进程进入后可抢占高队列,要正确输出进程调度过程,所有进程结束后输出甘特图,计算并输出响应时间、周转时间、等待时间等详细,给出c代码
时间: 2023-09-30 09:02:38 浏览: 29
以下是基于您所描述的调度策略的简单 C 代码,其中包含进程的创建、调度、计算和输出甘特图、响应时间、周转时间和等待时间。在这个模拟中,我们使用了一个结构体来表示进程和一个链表来存储它们。
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_TIME 100
#define MAX_PRIORITY 3
#define TIME_QUANTUM 4
typedef struct process {
int pid;
int arrival_time;
int burst_time;
int priority;
int remaining_time;
int start_time;
int end_time;
int response_time;
int turnaround_time;
int waiting_time;
struct process *next;
} Process;
Process *create_process(int pid, int arrival_time, int priority) {
Process *p = (Process *) malloc(sizeof(Process));
p->pid = pid;
p->arrival_time = arrival_time;
p->burst_time = rand() % 20 + 1; // 随机生成 1-20 的运行时间
p->priority = priority;
p->remaining_time = p->burst_time;
p->start_time = -1;
p->end_time = -1;
p->response_time = -1;
p->turnaround_time = -1;
p->waiting_time = 0;
p->next = NULL;
return p;
}
void print_gantt_chart(Process *head) {
printf("\n");
printf(" ");
for (int i = 0; i < MAX_TIME; i++) {
printf("--");
}
printf("\n|");
Process *p = head;
while (p != NULL) {
for (int i = p->start_time; i < p->end_time; i++) {
printf(" ");
}
printf("P%d", p->pid);
for (int i = p->start_time; i < p->end_time; i++) {
printf(" ");
}
printf("|");
p = p->next;
}
printf("\n ");
for (int i = 0; i < MAX_TIME; i++) {
printf("--");
}
printf("\n");
}
void calculate_metrics(Process *head) {
Process *p = head;
while (p != NULL) {
p->turnaround_time = p->end_time - p->arrival_time;
p->waiting_time = p->turnaround_time - p->burst_time;
p->response_time = p->start_time - p->arrival_time;
p = p->next;
}
}
void print_metrics(Process *head) {
Process *p = head;
printf("\n");
printf("PID AT BT PR ST ET RT TAT WT\n");
while (p != NULL) {
printf("%-4d %-3d %-3d %-3d %-3d %-3d %-3d %-3d %-3d\n", p->pid, p->arrival_time, p->burst_time,
p->priority, p->start_time, p->end_time, p->response_time, p->turnaround_time, p->waiting_time);
p = p->next;
}
}
int main() {
srand(time(NULL));
Process *head = NULL;
Process *tail = NULL;
int pid = 0;
for (int t = 0; t < MAX_TIME; t++) {
// 创建新进程
if (rand() % 5 == 0) { // 有 20% 的概率创建新进程
int priority = rand() % MAX_PRIORITY + 1;
Process *p = create_process(++pid, t, priority);
if (head == NULL) {
head = p;
} else {
tail->next = p;
}
tail = p;
printf("t=%d: Created process P%d (AT=%d, BT=%d, PR=%d)\n", t, p->pid, p->arrival_time, p->burst_time, p->priority);
}
// 选择进程
Process *current = NULL;
Process *prev = NULL;
for (int i = MAX_PRIORITY; i >= 1; i--) {
Process *p = head;
while (p != NULL) {
if (p->priority == i && p->remaining_time > 0 && p->arrival_time <= t) {
current = p;
break;
}
prev = p;
p = p->next;
}
if (current != NULL) {
break;
}
}
// 运行进程
if (current != NULL) {
if (current->start_time == -1) {
current->start_time = t;
current->response_time = t - current->arrival_time;
}
printf("t=%d: Running process P%d (PR=%d, RT=%d)\n", t, current->pid, current->priority, current->remaining_time);
current->remaining_time--;
if (current->remaining_time == 0) {
current->end_time = t + 1;
if (prev == NULL) {
head = current->next;
} else {
prev->next = current->next;
}
} else if (current->priority < MAX_PRIORITY && (t + 1) % TIME_QUANTUM == 0) {
// 降低优先级并移到队尾
current->priority++;
if (prev == NULL) {
head = current->next;
} else {
prev->next = current->next;
}
if (head == NULL) {
head = current;
} else {
tail->next = current;
}
tail = current;
}
} else {
printf("t=%d: CPU idle\n", t);
}
print_gantt_chart(head);
}
calculate_metrics(head);
print_metrics(head);
return 0;
}
```
请注意,此代码仅用于演示目的,可能需要根据实际需求进行更改和优化。
相关推荐
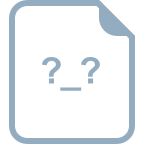















