springboot实现excel文件导入数据
时间: 2023-12-20 11:07:55 浏览: 25
在 Spring Boot 中,你可以使用 Apache POI 库来实现 Excel 文件的读取和导入数据。以下是一个简单的示例:
1. 添加 Maven 依赖
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建 Excel 文件模板
首先,你需要创建一个 Excel 文件模板,其中包含要导入的数据的列。
3. 创建 POJO 类
你需要创建一个 POJO 类来表示要导入的数据。该类的属性应该与 Excel 文件中的列相对应。
4. 编写读取 Excel 文件的代码
```java
@Service
public class ExcelService {
public List<YourPOJO> readExcel(MultipartFile file) throws IOException, InvalidFormatException {
Workbook workbook = WorkbookFactory.create(file.getInputStream());
Sheet sheet = workbook.getSheetAt(0);
List<YourPOJO> list = new ArrayList<>();
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
YourPOJO pojo = new YourPOJO();
pojo.setProp1(row.getCell(0).getStringCellValue());
pojo.setProp2(row.getCell(1).getStringCellValue());
// ...
list.add(pojo);
}
return list;
}
}
```
5. 编写控制器代码
```java
@RestController
public class YourController {
@Autowired
private ExcelService excelService;
@PostMapping("/import-excel")
public ResponseEntity<Void> importExcel(@RequestParam("file") MultipartFile file) throws IOException, InvalidFormatException {
List<YourPOJO> list = excelService.readExcel(file);
// 处理导入的数据
return ResponseEntity.ok().build();
}
}
```
这样,当你上传 Excel 文件时,它将被读取并转换为一个包含 YourPOJO 对象的列表,你可以使用它们来执行进一步的操作。
相关推荐
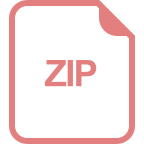
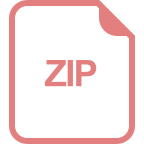
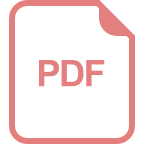














