import org.snmp4j.CommunityTarget;import org.snmp4j.PDU;import org.snmp4j.Snmp;import org.snmp4j.TransportMapping;import org.snmp4j.event.ResponseEvent;import org.snmp4j.mp.SnmpConstants;import org.snmp4j.smi.Address;import org.snmp4j.smi.GenericAddress;import org.snmp4j.smi.OID;import org.snmp4j.smi.VariableBinding;import org.snmp4j.transport.DefaultUdpTransportMapping;public class HuaweiRouterSNMP { public static void main(String[] args) { // 设备IP地址 String ipAddress = "192.168.1.1"; // SNMP社区名 String community = "public"; // 设备OID String oidValue = "1.3.6.1.2.1.1.1.0"; try { // 创建SNMP对象 TransportMapping transport = new DefaultUdpTransportMapping(); Snmp snmp = new Snmp(transport); transport.listen(); // 创建目标对象 Address targetAddress = GenericAddress.parse("udp:" + ipAddress + "/161"); CommunityTarget target = new CommunityTarget(); target.setCommunity(GenericAddress.parse(community)); target.setAddress(targetAddress); target.setRetries(2); target.setTimeout(5000); target.setVersion(SnmpConstants.version2c); // 创建PDU对象 PDU pdu = new PDU(); pdu.add(new VariableBinding(new OID(oidValue))); pdu.setType(PDU.GET); // 发送请求 ResponseEvent response = snmp.send(pdu, target); if (response.getResponse() != null) { System.out.println("Response: " + response.getResponse().get(0).getVariable().toString()); } else { System.out.println("Error: " + response.getError()); } // 关闭SNMP连接 snmp.close(); } catch (Exception e) { e.printStackTrace(); } }}查看此段代码是否有错
时间: 2024-02-06 18:04:18 浏览: 80
这段代码看起来基本上是可以工作的,但是需要注意以下几点:
1. `target.setCommunity(GenericAddress.parse(community));` 应该改为 `target.setCommunity(new OctetString(community));`,因为 `setCommunity` 的参数类型是 `OctetString`。
2. `target.setVersion(SnmpConstants.version2c);` 指定的是 SNMP v2c 版本,如果你想要使用 SNMP v1 版本,应该将其改为 `target.setVersion(SnmpConstants.version1);`。
3. SNMP 返回的结果是一个数组,即使你只请求一个 OID,也需要使用 `response.getResponse().get(0)` 获取结果。
4. 为了避免出现 `java.net.BindException: Address already in use: Cannot bind` 错误,你应该在程序结束时关闭 `transport`。
下面是修正后的代码:
```java
import org.snmp4j.CommunityTarget;
import org.snmp4j.PDU;
import org.snmp4j.Snmp;
import org.snmp4j.TransportMapping;
import org.snmp4j.event.ResponseEvent;
import org.snmp4j.mp.SnmpConstants;
import org.snmp4j.smi.Address;
import org.snmp4j.smi.GenericAddress;
import org.snmp4j.smi.OID;
import org.snmp4j.smi.OctetString;
import org.snmp4j.smi.VariableBinding;
import org.snmp4j.transport.DefaultUdpTransportMapping;
public class HuaweiRouterSNMP {
public static void main(String[] args) {
// 设备IP地址
String ipAddress = "192.168.1.1";
// SNMP社区名
String community = "public";
// 设备OID
String oidValue = "1.3.6.1.2.1.1.1.0";
try {
// 创建SNMP对象
TransportMapping<? extends Address> transport = new DefaultUdpTransportMapping();
Snmp snmp = new Snmp(transport);
transport.listen();
// 创建目标对象
Address targetAddress = GenericAddress.parse("udp:" + ipAddress + "/161");
CommunityTarget target = new CommunityTarget();
target.setCommunity(new OctetString(community));
target.setAddress(targetAddress);
target.setRetries(2);
target.setTimeout(5000);
target.setVersion(SnmpConstants.version2c);
// 创建PDU对象
PDU pdu = new PDU();
pdu.add(new VariableBinding(new OID(oidValue)));
pdu.setType(PDU.GET);
// 发送请求
ResponseEvent response = snmp.send(pdu, target);
if (response.getResponse() != null) {
System.out.println("Response: " + response.getResponse().get(0));
} else {
System.out.println("Error: " + response.getError());
}
// 关闭SNMP连接
snmp.close();
transport.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
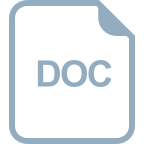
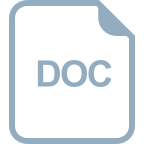
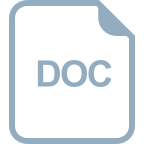

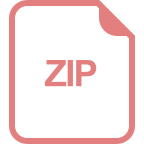
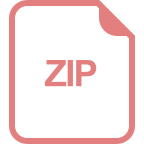
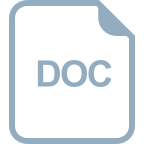
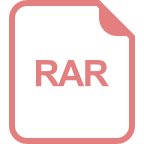
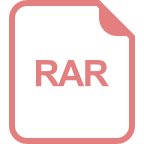
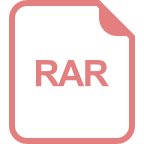
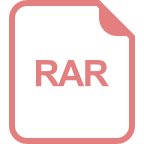
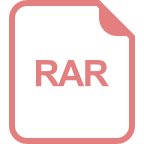
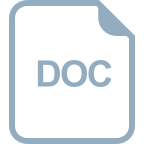
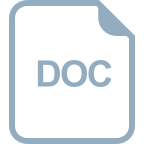




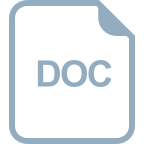
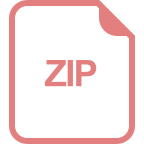