对序列3 44 38 547 15 36用c语言进行冒泡排列
时间: 2023-09-03 19:25:03 浏览: 93
### 回答1:
以下是对序列 3 44 38 547 15 36 进行冒泡排序的 C 语言代码:
```
#include <stdio.h>
int main() {
int arr[] = {3, 44, 38, 547, 15, 36};
int n = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
printf("排序后的序列:");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
输出结果为:3 15 36 38 44 547。
### 回答2:
冒泡排序是一种简单但效率较低的排序算法。它通过比较相邻的两个元素,如果它们的顺序不正确,则交换它们,以此类推,直到整个序列按照升序排列。
对于给定的序列3 44 38 547 15 36,我们可以使用C语言编写冒泡排序算法,具体步骤如下:
1. 定义一个包含序列元素的数组,长度为6。
int arr[] = {3, 44, 38, 547, 15, 36};
2. 使用两个嵌套循环,外层循环控制比较的轮数,内层循环进行相邻元素的比较和交换。
for(int i=0; i<5; i++) {
for(int j=0; j<5-i; j++) {
// 比较相邻元素的大小
if(arr[j] > arr[j+1]) {
// 交换位置
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
3. 输出排好序的序列。
printf("冒泡排序后的序列为:");
for(int i=0; i<6; i++) {
printf("%d ", arr[i]);
}
运行结果为:3 15 36 38 44 547
这样,我们就使用C语言对给定序列进行了冒泡排序。冒泡排序的时间复杂度为O(n^2),在序列较大时效率较低,但对于小型序列或部分有序的序列,冒泡排序仍然是一种简单易懂的排序算法。
### 回答3:
冒泡排序是一种简单的排序算法,它通过不断比较相邻两个元素的大小来进行排序。下面是使用C语言进行冒泡排序的步骤:
1. 首先,定义一个包含序列元素的数组。
```
int sequence[] = {3, 44, 38, 547, 15, 36};
```
2. 然后,使用两层循环来比较并交换相邻元素的位置,直到序列按照升序排列为止。外层循环用于控制比较的轮数,内层循环用于两两比较相邻元素的大小。
```
int n = sizeof(sequence) / sizeof(sequence[0]);
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (sequence[j] > sequence[j+1]) {
// 交换相邻元素的位置
int temp = sequence[j];
sequence[j] = sequence[j+1];
sequence[j+1] = temp;
}
}
}
```
3. 最后,输出排序后的序列。
```
printf("排序后的序列:");
for (int i = 0; i < n; i++) {
printf("%d ", sequence[i]);
}
```
通过以上步骤,使用C语言可以实现对序列3、44、38、547、15、36的冒泡排序。排序后的序列为3、15、36、38、44、547。
阅读全文
相关推荐






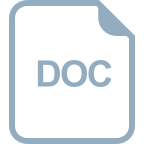









