MFC如何将多张图片上传到 http://169.254.1.10:8090/api/v1/~bali/ABC0123456789
时间: 2023-10-05 07:06:14 浏览: 81
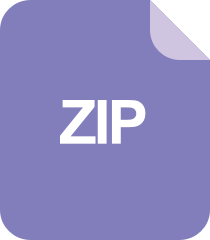
多张图片上传到服务器
您可以按照以下步骤实现:
1. 打开文件对话框,让用户选择需要上传的图片文件,可以使用 CFileDialog 类实现。
2. 将选择的文件路径保存到一个字符串数组中。
3. 使用 WinHTTP API 发送 HTTP POST 请求,并将文件作为二进制数据附加到请求中。可以使用 CHttpFile 类实现。
4. 在请求头中添加必要的信息,例如 Content-Type、Content-Length 等。
5. 在请求体中添加每个文件的二进制数据,并在每个文件数据前添加一个分隔符。
6. 发送请求并等待响应,处理响应结果。
下面是一个简单的示例代码,仅供参考:
```cpp
void CMyDlg::OnUploadBtnClicked()
{
// 打开文件对话框,选择需要上传的图片文件
CStringArray arrFileNames;
CFileDialog dlg(TRUE, NULL, NULL, OFN_ALLOWMULTISELECT | OFN_FILEMUSTEXIST,
_T("图片文件 (*.bmp;*.jpg;*.jpeg;*.png)|*.bmp;*.jpg;*.jpeg;*.png||"), this);
dlg.m_ofn.lpstrFile = new TCHAR[MAX_PATH * 100];
dlg.m_ofn.nMaxFile = MAX_PATH * 100;
if (dlg.DoModal() == IDOK)
{
POSITION pos = dlg.GetStartPosition();
while (pos != NULL)
{
CString strFileName = dlg.GetNextPathName(pos);
arrFileNames.Add(strFileName);
}
}
delete[] dlg.m_ofn.lpstrFile;
// 发送 HTTP POST 请求,上传图片文件
CString strBoundary = _T("----MyBoundary1234567890");
CString strContentType = _T("multipart/form-data; boundary=") + strBoundary;
CString strHost = _T("169.254.1.10");
CString strUrl = _T("/api/v1/~bali/ABC0123456789");
CInternetSession session;
CHttpConnection* pConnection = session.GetHttpConnection(strHost);
if (pConnection != NULL)
{
CString strHeaders = _T("Content-Type: ") + strContentType + _T("\r\n");
CHttpFile* pFile = pConnection->OpenRequest(CHttpConnection::HTTP_VERB_POST, strUrl, NULL, 1, NULL, NULL, INTERNET_FLAG_DONT_CACHE);
if (pFile != NULL)
{
// 添加请求头
pFile->AddRequestHeaders(strHeaders, HTTP_ADDREQ_FLAG_REPLACE);
// 计算请求体长度
ULONGLONG nContentLength = CalcRequestContentLength(arrFileNames, strBoundary);
// 添加请求体
if (pFile->SendRequestEx(nContentLength, HSR_SYNC | HSR_INITIATE))
{
AddRequestContent(arrFileNames, strBoundary, pFile);
pFile->EndRequest(HSR_SYNC | HSR_TERMINATE);
// 处理响应结果
DWORD dwStatusCode;
pFile->QueryInfoStatusCode(dwStatusCode);
if (dwStatusCode == HTTP_STATUS_OK)
{
// 上传成功
AfxMessageBox(_T("上传成功!"));
}
else
{
// 上传失败
AfxMessageBox(_T("上传失败!"));
}
}
else
{
// 发送请求失败
AfxMessageBox(_T("发送请求失败!"));
}
pFile->Close();
delete pFile;
}
else
{
// 打开请求失败
AfxMessageBox(_T("打开请求失败!"));
}
pConnection->Close();
delete pConnection;
}
else
{
// 建立连接失败
AfxMessageBox(_T("建立连接失败!"));
}
}
ULONGLONG CMyDlg::CalcRequestContentLength(const CStringArray& arrFileNames, const CString& strBoundary)
{
ULONGLONG nLength = 0;
// 计算每个文件的长度
for (int i = 0; i < arrFileNames.GetSize(); i++)
{
CString strFileName = arrFileNames[i];
CFile file;
if (file.Open(strFileName, CFile::modeRead | CFile::shareDenyWrite))
{
nLength += strBoundary.GetLength() + 2; // 添加分隔符和换行符
nLength += GetFileContentLength(&file); // 添加文件内容长度
nLength += 2; // 添加换行符
file.Close();
}
}
// 添加结束分隔符和换行符
nLength += strBoundary.GetLength() + 2 + 2;
return nLength;
}
ULONGLONG CMyDlg::GetFileContentLength(CFile* pFile)
{
ULONGLONG nLength = 0;
if (pFile != NULL)
{
nLength = pFile->GetLength();
}
return nLength;
}
void CMyDlg::AddRequestContent(const CStringArray& arrFileNames, const CString& strBoundary, CHttpFile* pFile)
{
// 添加每个文件的内容
for (int i = 0; i < arrFileNames.GetSize(); i++)
{
CString strFileName = arrFileNames[i];
CFile file;
if (file.Open(strFileName, CFile::modeRead | CFile::shareDenyWrite))
{
CString strContentDisposition = _T("Content-Disposition: form-data; name=\"file\"; filename=\"") + GetFileName(strFileName) + _T("\"\r\n");
CString strContentType = _T("Content-Type: application/octet-stream\r\n");
CString strContentLengthHeader = _T("Content-Length: ") + FormatFileSize(GetFileContentLength(&file)) + _T("\r\n");
CString strCRLF = _T("\r\n");
// 添加分隔符和请求头
pFile->Write((LPCTSTR)strBoundary, strBoundary.GetLength());
pFile->Write(_T("\r\n"), 2);
pFile->Write((LPCTSTR)strContentDisposition, strContentDisposition.GetLength());
pFile->Write((LPCTSTR)strContentType, strContentType.GetLength());
pFile->Write((LPCTSTR)strContentLengthHeader, strContentLengthHeader.GetLength());
pFile->Write((LPCTSTR)strCRLF, strCRLF.GetLength());
// 添加文件内容
BYTE buffer[4096];
UINT nBytesRead;
while ((nBytesRead = file.Read(buffer, 4096)) > 0)
{
pFile->Write(buffer, nBytesRead);
}
// 添加换行符
pFile->Write((LPCTSTR)strCRLF, strCRLF.GetLength());
file.Close();
}
}
// 添加结束分隔符和换行符
CString strEndBoundary = strBoundary + _T("--");
pFile->Write((LPCTSTR)strEndBoundary, strEndBoundary.GetLength());
pFile->Write(_T("\r\n"), 2);
}
CString CMyDlg::GetFileName(const CString& strPath)
{
int nPos = strPath.ReverseFind(_T('\\'));
if (nPos != -1)
{
return strPath.Mid(nPos + 1);
}
else
{
return strPath;
}
}
CString CMyDlg::FormatFileSize(ULONGLONG nFileSize)
{
CString strFileSize;
if (nFileSize >= 1024 * 1024 * 1024)
{
strFileSize.Format(_T("%.2f GB"), (double)nFileSize / (1024 * 1024 * 1024));
}
else if (nFileSize >= 1024 * 1024)
{
strFileSize.Format(_T("%.2f MB"), (double)nFileSize / (1024 * 1024));
}
else if (nFileSize >= 1024)
{
strFileSize.Format(_T("%.2f KB"), (double)nFileSize / 1024);
}
else
{
strFileSize.Format(_T("%d bytes"), nFileSize);
}
return strFileSize;
}
```
阅读全文
相关推荐














