java实现滑动图片拼图验证
时间: 2023-10-05 20:12:54 浏览: 109
实现滑动图片拼图验证的步骤如下:
1.准备两张图片,一张是完整的原图,另一张是被拆分的拼图。
2.使用Java的Graphics类将原图和拼图绘制到JPanel上。
3.使用Java的Random类生成一个随机位置,将拼图绘制到原图的随机位置。
4.使用Java的MouseAdapter类监听鼠标事件,当用户按下鼠标并拖动时,将拼图跟随鼠标移动。
5.使用Java的Rectangle类判断拼图是否在原图的正确位置,如果是,则验证通过,否则提示用户重新拼图。
下面是一个简单的Java代码示例:
```java
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.util.Random;
import javax.swing.*;
public class SlidePuzzle extends JPanel {
private Image img; // 原图
private Image puzzleImg; // 拼图
private Point puzzleLoc; // 拼图位置
private Rectangle puzzleRect; // 拼图矩形
private boolean isDragging; // 是否正在拖动
public SlidePuzzle() {
// 加载图片
img = new ImageIcon("original.jpg").getImage();
puzzleImg = new ImageIcon("puzzle.jpg").getImage();
// 随机生成拼图位置
Random rand = new Random();
int x = rand.nextInt(img.getWidth(null) - puzzleImg.getWidth(null));
int y = rand.nextInt(img.getHeight(null) - puzzleImg.getHeight(null));
puzzleLoc = new Point(x, y);
// 创建拼图矩形
puzzleRect = new Rectangle(puzzleLoc.x, puzzleLoc.y, puzzleImg.getWidth(null), puzzleImg.getHeight(null));
// 添加鼠标监听器
addMouseListener(new MouseAdapter() {
public void mousePressed(MouseEvent e) {
if (puzzleRect.contains(e.getPoint())) {
isDragging = true;
}
}
public void mouseReleased(MouseEvent e) {
if (isDragging) {
isDragging = false;
if (puzzleRect.intersects(new Rectangle(300, 300, 100, 100))) {
JOptionPane.showMessageDialog(null, "验证通过!");
} else {
JOptionPane.showMessageDialog(null, "拼图不正确,请重新拼图!");
}
}
}
});
addMouseMotionListener(new MouseAdapter() {
public void mouseDragged(MouseEvent e) {
if (isDragging) {
puzzleLoc.translate(e.getX() - puzzleRect.x, e.getY() - puzzleRect.y);
puzzleRect.setLocation(puzzleLoc);
repaint();
}
}
});
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(img, 0, 0, null);
g.drawImage(puzzleImg, puzzleLoc.x, puzzleLoc.y, null);
}
public static void main(String[] args) {
JFrame frame = new JFrame("滑动拼图验证");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(600, 600);
frame.add(new SlidePuzzle());
frame.setVisible(true);
}
}
```
阅读全文
相关推荐




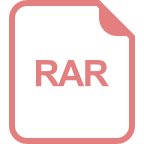









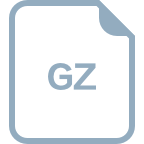

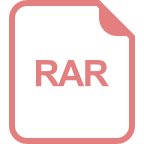