使用Java绘制游戏战力能力项多边形蛛网图,每个能力项相连绘制一张图含有透明的颜色背景,并输出为图片
时间: 2024-04-04 12:33:58 浏览: 14
好的,我可以为您提供一些思路和代码示例。首先,您可以使用Java的Graphics2D类来绘制多边形蛛网图和背景图。以下是一个简单的示例代码:
```java
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class SpiderChart {
public static void main(String[] args) {
int width = 500;
int height = 500;
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D g = image.createGraphics();
// 绘制多边形蛛网图
int cx = width / 2;
int cy = height / 2;
int r = Math.min(cx, cy) - 10;
int n = 6; // 边数
double angle = 2 * Math.PI / n;
int[] x = new int[n];
int[] y = new int[n];
for (int i = 0; i < n; i++) {
x[i] = (int) (cx + r * Math.sin(i * angle));
y[i] = (int) (cy - r * Math.cos(i * angle));
}
g.setColor(Color.BLACK);
g.drawPolygon(x, y, n);
for (int i = 1; i < n; i++) {
g.drawLine(cx, cy, x[i], y[i]);
}
// 绘制背景图
BufferedImage bgImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D bg = bgImage.createGraphics();
bg.setColor(new Color(255, 0, 0, 128)); // 红色透明背景
bg.fillRect(0, 0, width, height);
// 合并两张图
g.drawImage(bgImage, 0, 0, null);
// 输出为图片
try {
ImageIO.write(image, "png", new File("spider_chart.png"));
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上代码会生成一个大小为500x500的多边形蛛网图,背景为红色半透明,输出为PNG格式的图片。您可以根据自己的需求修改代码中的参数和绘制方式。
相关推荐
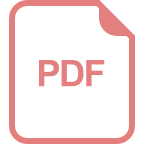










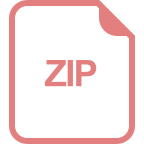
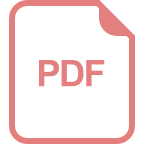