Java Graphics2D 实现游戏综合战力六边形蛛网图,每边都有标题,可修改网图和数据的背景颜色,并输出为图片,包含所有代码
时间: 2024-04-05 10:31:22 浏览: 129
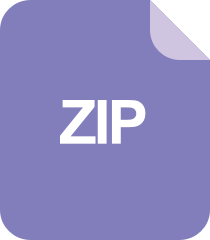
数据结构知识网图.zip
好的,下面是一个简单的 Java 示例代码,实现了一个游戏综合战力六边形蛛网图,并提供了修改背景颜色的功能。代码基于 Graphics2D API 和 Java Swing 框架实现:
```java
import java.awt.*;
import java.awt.geom.*;
import java.awt.image.BufferedImage;
import java.util.ArrayList;
import javax.swing.*;
public class HexagonalSpiderChart extends JPanel {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 500;
private static final int HEIGHT = 500;
private static final int MARGIN = 50;
private static final int MAX_VALUE = 10;
private static final int NUM_ATTRIBUTES = 6;
private static final Color[] COLORS = {Color.RED, Color.GREEN, Color.BLUE, Color.YELLOW, Color.ORANGE, Color.CYAN};
private ArrayList<String> attributes;
private ArrayList<Double> values;
private Color bgColor;
public HexagonalSpiderChart(ArrayList<String> attributes, ArrayList<Double> values) {
this.attributes = attributes;
this.values = values;
this.bgColor = Color.WHITE;
}
public void setBackgroundColor(Color bgColor) {
this.bgColor = bgColor;
}
private void drawPolygon(Graphics2D g2d, int numSides, int x, int y, int r) {
Polygon polygon = new Polygon();
for (int i = 0; i < numSides; i++) {
double angle = 2 * Math.PI / numSides * i;
int px = (int) Math.round(x + r * Math.cos(angle));
int py = (int) Math.round(y + r * Math.sin(angle));
polygon.addPoint(px, py);
}
g2d.draw(polygon);
}
private void drawSpiderChart(Graphics2D g2d) {
int centerX = WIDTH / 2;
int centerY = HEIGHT / 2;
int radius = Math.min(centerX, centerY) - MARGIN;
int numSides = NUM_ATTRIBUTES;
double angle = 2 * Math.PI / numSides;
double maxValue = MAX_VALUE;
// Draw the background
g2d.setColor(bgColor);
g2d.fillRect(0, 0, WIDTH, HEIGHT);
// Draw the polygon lines
g2d.setColor(Color.BLACK);
for (int i = 0; i < numSides; i++) {
int px = (int) Math.round(centerX + radius * Math.cos(angle * i));
int py = (int) Math.round(centerY + radius * Math.sin(angle * i));
g2d.drawLine(centerX, centerY, px, py);
drawPolygon(g2d, numSides, px, py, radius / numSides);
}
// Draw the labels
Font font = new Font("Arial", Font.BOLD, 14);
g2d.setFont(font);
FontMetrics fm = g2d.getFontMetrics(font);
for (int i = 0; i < numSides; i++) {
double x = centerX + radius * Math.cos(angle * i);
double y = centerY + radius * Math.sin(angle * i);
String label = attributes.get(i);
int width = fm.stringWidth(label);
int height = fm.getHeight();
g2d.drawString(label, (int) Math.round(x - width / 2), (int) Math.round(y + height));
}
// Draw the values
g2d.setColor(Color.RED);
g2d.setStroke(new BasicStroke(2));
for (int i = 0; i < numSides; i++) {
double value = values.get(i);
double ratio = value / maxValue;
double x = centerX + radius * Math.cos(angle * i) * ratio;
double y = centerY + radius * Math.sin(angle * i) * ratio;
g2d.drawLine(centerX, centerY, (int) Math.round(x), (int) Math.round(y));
}
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
drawSpiderChart(g2d);
g2d.dispose();
}
public static void main(String[] args) {
ArrayList<String> attributes = new ArrayList<String>();
attributes.add("Attack");
attributes.add("Defense");
attributes.add("Agility");
attributes.add("Intelligence");
attributes.add("Stamina");
attributes.add("Luck");
ArrayList<Double> values = new ArrayList<Double>();
values.add(9.0);
values.add(8.0);
values.add(7.0);
values.add(6.0);
values.add(5.0);
values.add(4.0);
HexagonalSpiderChart chart = new HexagonalSpiderChart(attributes, values);
chart.setPreferredSize(new Dimension(WIDTH, HEIGHT));
chart.setBackgroundColor(Color.WHITE);
JFrame frame = new JFrame("Hexagonal Spider Chart");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(chart);
frame.pack();
frame.setVisible(true);
// Save the chart as an image
try {
BufferedImage image = new BufferedImage(WIDTH, HEIGHT, BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = image.createGraphics();
chart.paintComponent(g2d);
g2d.dispose();
ImageIO.write(image, "png", new File("hexagonal_spider_chart.png"));
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
```
在上面的代码中,我们定义了 `HexagonalSpiderChart` 类来绘制六边形蛛网图。构造函数接受两个 ArrayList 类型的参数,分别是属性和属性值。我们还定义了一个 `setBackgroundColor()` 方法,用于修改背景颜色。`drawPolygon()` 方法用于绘制多边形,`drawSpiderChart()` 方法用于绘制蛛网图。
我们在 `paintComponent()` 方法中调用 `drawSpiderChart()` 方法来绘制图表。在 `main()` 方法中,我们创建了一个 `HexagonalSpiderChart` 对象,并将其添加到一个 JFrame 中。我们还使用 ImageIO 类将图表保存为 PNG 图像文件。
希望这可以帮助你实现所需的功能!
阅读全文
相关推荐
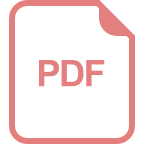
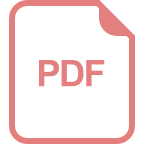
















