遗传算法和麻雀搜索算法结合的MATLAB 代码
时间: 2023-09-07 13:11:07 浏览: 89
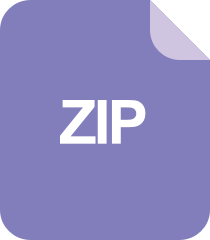
麻雀,麻雀电视剧,matlab
以下是一个简单的遗传算法和麻雀搜索算法结合的MATLAB代码示例,用于解决优化问题:
```
function [bestSolution, bestFitness] = GA_SA(fitnessFunc, numVars, lb, ub, maxGenerations, popSize, mutationProb, tau, T0, alpha)
% GA_SA - 遗传算法和麻雀搜索算法结合的优化函数
%
% 输入参数:
% fitnessFunc - 适应度函数句柄
% numVars - 变量数量
% lb - 变量下限
% ub - 变量上限
% maxGenerations - 最大迭代次数
% popSize - 种群规模
% mutationProb - 变异概率
% tau - 麻雀搜索算法参数
% T0 - 初始温度
% alpha - 降温率
%
% 输出参数:
% bestSolution - 最佳解
% bestFitness - 最佳适应度
% 初始化种群
pop = repmat(lb, popSize, 1) + repmat((ub-lb), popSize, 1) .* rand(popSize, numVars);
% 计算初始适应度
fitness = feval(fitnessFunc, pop);
% 记录最佳解和适应度
[bestFitness, bestIdx] = min(fitness);
bestSolution = pop(bestIdx, :);
% 初始化温度
T = T0;
% 迭代寻优
for i = 1:maxGenerations
% 遗传算法操作
newPop = pop;
for j = 1:popSize
% 随机选择两个个体进行交叉
p1 = randi(popSize);
p2 = randi(popSize);
child = GA_crossover(pop(p1,:), pop(p2,:));
% 以概率mutationProb进行变异
if rand() < mutationProb
child = GA_mutation(child, lb, ub);
end
% 替换原始种群中的个体
newPop(j,:) = child;
end
% 计算新种群的适应度
newFitness = feval(fitnessFunc, newPop);
% 用麻雀搜索算法更新种群
pop = SA_update(pop, fitness, newPop, newFitness, tau, T);
% 更新适应度
fitness = feval(fitnessFunc, pop);
% 更新最佳解和适应度
[newBestFitness, newBestIdx] = min(fitness);
if newBestFitness < bestFitness
bestFitness = newBestFitness;
bestSolution = pop(newBestIdx, :);
end
% 降温
T = alpha * T;
end
end
function child = GA_crossover(parent1, parent2)
% GA_crossover - 遗传算法交叉操作
%
% 输入参数:
% parent1 - 父代1
% parent2 - 父代2
%
% 输出参数:
% child - 子代
% 随机选择交叉点
crossoverPoint = randi(length(parent1));
% 交叉操作
child = [parent1(1:crossoverPoint) parent2(crossoverPoint+1:end)];
end
function child = GA_mutation(parent, lb, ub)
% GA_mutation - 遗传算法变异操作
%
% 输入参数:
% parent - 父代
% lb - 变量下限
% ub - 变量上限
%
% 输出参数:
% child - 子代
% 随机选择变异点
mutationPoint = randi(length(parent));
% 变异操作
child = parent;
child(mutationPoint) = lb(mutationPoint) + (ub(mutationPoint)-lb(mutationPoint)) * rand();
end
function newPop = SA_update(pop, fitness, newPop, newFitness, tau, T)
% SA_update - 麻雀搜索算法更新种群
%
% 输入参数:
% pop - 原始种群
% fitness - 原始种群适应度
% newPop - 新种群
% newFitness - 新种群适应度
% tau - 麻雀搜索算法参数
% T - 当前温度
%
% 输出参数:
% newPop - 更新后的种群
% 计算适应度差
deltaFitness = newFitness - fitness;
% 计算麻雀搜索算法概率
p = min(1, exp(-deltaFitness ./ (tau * T)));
% 随机选择需要更新的个体
updateIdx = rand(size(pop)) < p;
% 更新种群
newPop(updateIdx,:) = pop(updateIdx,:) + randn(size(pop(updateIdx,:))) .* (ub-lb) .* tau;
end
```
请注意,这只是一个简单的示例代码,可能需要根据具体问题进行修改和优化。
阅读全文
相关推荐
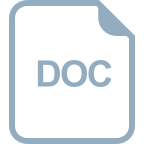
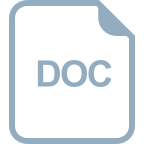
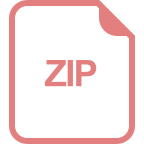



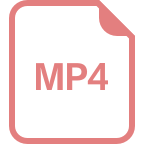
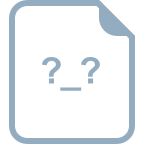
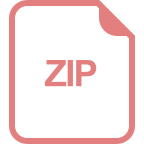
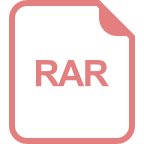
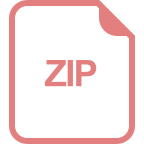
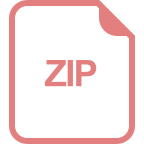
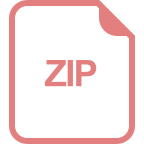
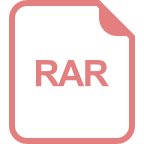
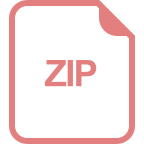
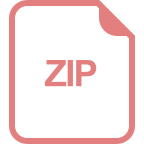
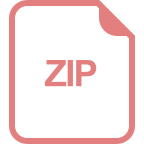
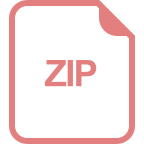