python写五子棋人机对战
时间: 2023-10-01 09:05:32 浏览: 103
好的,下面是一个简单的五子棋人机对战的实现,使用 Python 语言和 Pygame 库。
首先,需要安装 Pygame 库,可以使用以下命令安装:
```
pip install pygame
```
接下来,我们需要创建一个游戏窗口,初始化 Pygame 库并设置一些基本参数。
```python
import pygame
# 初始化 Pygame 库
pygame.init()
# 设置游戏窗口大小和标题
WINDOW_SIZE = (640, 640)
window = pygame.display.set_mode(WINDOW_SIZE)
pygame.display.set_caption("五子棋人机对战")
# 设置棋盘大小和格子大小
BOARD_SIZE = 15
GRID_SIZE = WINDOW_SIZE[0] // BOARD_SIZE
# 设置棋子半径
PIECE_RADIUS = GRID_SIZE // 2 - 2
# 设置棋子颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
```
接下来,我们需要实现棋盘和棋子的绘制。在绘制棋盘时,需要先绘制背景色,然后绘制棋盘网格,最后绘制棋盘上的星点。在绘制棋子时,需要根据棋子的颜色绘制不同的圆形。
```python
# 绘制棋盘和棋子
def draw_board(board):
# 绘制背景色
window.fill((200, 160, 60))
# 绘制棋盘网格
for i in range(BOARD_SIZE):
pygame.draw.line(window, BLACK, (GRID_SIZE, (i + 1) * GRID_SIZE), (WINDOW_SIZE[0] - GRID_SIZE, (i + 1) * GRID_SIZE), 1)
pygame.draw.line(window, BLACK, ((i + 1) * GRID_SIZE, GRID_SIZE), ((i + 1) * GRID_SIZE, WINDOW_SIZE[1] - GRID_SIZE), 1)
# 绘制棋盘上的星点
for i in range(3):
for j in range(3):
if i == 1 and j == 1:
pygame.draw.circle(window, BLACK, (GRID_SIZE * 8, GRID_SIZE * 8), 5)
else:
pygame.draw.circle(window, BLACK, (GRID_SIZE * (3 + 4 * i), GRID_SIZE * (3 + 4 * j)), 5)
# 绘制棋子
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == 1:
pygame.draw.circle(window, BLACK, ((j + 1) * GRID_SIZE, (i + 1) * GRID_SIZE), PIECE_RADIUS)
elif board[i][j] == 2:
pygame.draw.circle(window, WHITE, ((j + 1) * GRID_SIZE, (i + 1) * GRID_SIZE), PIECE_RADIUS)
```
接下来,我们需要实现落子和判断胜利的功能。在落子时,需要判断当前落子的位置是否合法,如果合法则将棋子放置在相应的位置上。在判断胜利时,需要遍历棋盘上的所有棋子,对于每个棋子,判断其周围的相同颜色的棋子是否构成了五子连珠,如果是则返回当前棋子的颜色。
```python
# 落子
def place_piece(board, row, col, player):
if board[row][col] == 0:
board[row][col] = player
return True
return False
# 判断胜利
def check_win(board):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] != 0:
for dx, dy in [(0, 1), (1, 0), (1, 1), (1, -1)]:
x, y = i, j
count = 0
while x >= 0 and x < BOARD_SIZE and y >= 0 and y < BOARD_SIZE and board[x][y] == board[i][j]:
count += 1
x += dx
y += dy
if count >= 5:
return board[i][j]
return 0
```
最后,我们需要实现人机对战的主循环。在每次循环中,需要检测用户输入,如果用户点击了鼠标,则根据鼠标的位置计算出落子的位置,并落下相应的棋子。然后,需要判断是否有人胜利,如果有则输出胜利信息并退出程序。如果没有胜利,则切换玩家并进行下一轮。
```python
# 人机对战的主循环
def main():
# 初始化棋盘
board = [[0] * BOARD_SIZE for i in range(BOARD_SIZE)]
# 设置当前玩家
current_player = 1
# 主循环
while True:
# 处理用户输入
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if current_player == 1:
x, y = event.pos
row, col = y // GRID_SIZE - 1, x // GRID_SIZE - 1
if place_piece(board, row, col, current_player):
current_player = 2
# 绘制棋盘和棋子
draw_board(board)
pygame.display.update()
# 判断是否有人胜利
winner = check_win(board)
if winner == 1:
print("黑方胜利!")
pygame.quit()
exit()
elif winner == 2:
print("白方胜利!")
pygame.quit()
exit()
# 切换玩家
if current_player == 2:
row, col = ai_move(board)
place_piece(board, row, col, current_player)
current_player = 1
```
其中,ai_move 函数是人机对战中 AI 落子的函数,需要根据当前棋盘状态和当前玩家的颜色计算出最优的落子位置。这里我们使用了一个简单的随机算法实现。
```python
# AI 落子
def ai_move(board):
# 随机落子
import random
row, col = random.randint(0, BOARD_SIZE - 1), random.randint(0, BOARD_SIZE - 1)
while board[row][col] != 0:
row, col = random.randint(0, BOARD_SIZE - 1), random.randint(0, BOARD_SIZE - 1)
return row, col
```
最后,调用 main 函数即可开始人机对战。
```python
if __name__ == '__main__':
main()
```
阅读全文
相关推荐
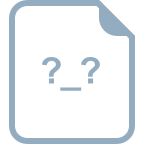
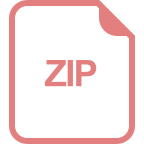



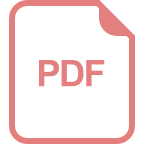
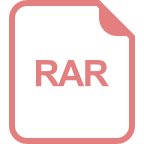
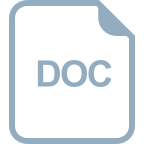
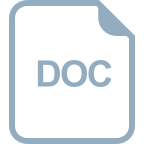





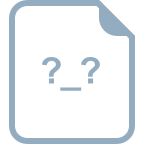