写一段java通过数据库查询是否还有剩余次数的代码
时间: 2023-09-24 11:06:27 浏览: 87
### 回答1:
以下是一段示例代码:
```
import java.sql.*;
public class CheckRemaining {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
// 加载驱动
Class.forName("com.mysql.jdbc.Driver");
// 建立数据库连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "username", "password");
// 创建SQL语句
String sql = "SELECT remaining FROM table WHERE user_id = ?";
// 预处理语句
stmt = conn.prepareStatement(sql);
stmt.setInt(1, 12345);
// 执行查询
rs = stmt.executeQuery();
if (rs.next()) {
int remaining = rs.getInt("remaining");
if (remaining > 0) {
System.out.println("还有剩余次数");
} else {
System.out.println("没有剩余次数");
}
}
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
该代码首先加载MySQL数据库的驱动,然后连接数据库并创建预处理语句,并通过该语句执行查询。如果结果集中有数据,则检查剩余次数是否大于零,并作出相应的输出。最后,该代码关闭了数据库连接。
### 回答2:
确定理解你的问题正确的话,请注意以下简短的代码段。这段代码使用Java的JDBC连接数据库,并查询指定表中的剩余次数列。查询结果以布尔形式返回,表示是否还有剩余次数。
```java
import java.sql.*;
public class CheckRemainingCount {
public static void main(String[] args) {
// 数据库连接信息
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "root";
String password = "password";
// 执行查询的SQL语句
String query = "SELECT remaining_count FROM mytable";
// 尝试连接数据库并查询结果
try (Connection connection = DriverManager.getConnection(url, username, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(query)) {
// 判断查询结果是否为空
boolean hasRemainingCount = resultSet.next();
if (hasRemainingCount) {
// 获取剩余次数列的值
int remainingCount = resultSet.getInt("remaining_count");
// 根据剩余次数的值进行相应的处理
if (remainingCount > 0) {
System.out.println("还有剩余次数");
} else {
System.out.println("没有剩余次数");
}
} else {
System.out.println("查询结果为空");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
这段代码中的`url`、`username`和`password`需要根据你自己的数据库连接信息进行修改。`query`需要根据你的实际需求修改为对应的SQL查询语句。另外,需要确保你的Java项目中已经引入了适当的JDBC驱动程序。更详细的数据库连接和查询操作可以参考Java的JDBC文档。
相关推荐
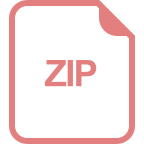
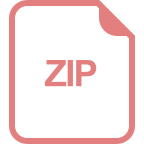
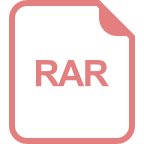











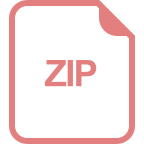
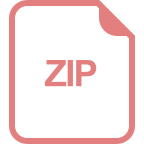
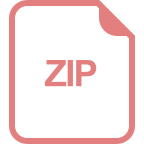
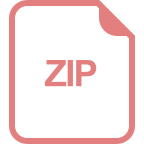