Vue封装下拉刷新组件
时间: 2023-08-30 14:06:11 浏览: 148
好的,下面是一个简单的Vue下拉刷新组件的封装示例:
```vue
<template>
<div class="pull-to-refresh" ref="wrapper">
<div class="pull-to-refresh__icon">
<i :class="iconClass"></i>
</div>
<div class="pull-to-refresh__text">{{text}}</div>
<div class="pull-to-refresh__content">
<slot></slot>
</div>
</div>
</template>
<script>
export default {
name: 'PullToRefresh',
props: {
iconClass: {
type: String,
default: 'iconfont icon-refresh'
},
text: {
type: String,
default: '下拉刷新'
}
},
data() {
return {
startY: 0,
moveY: 0,
distance: 0,
isDragging: false
}
},
mounted() {
this.$refs.wrapper.addEventListener('touchstart', this.handleTouchStart)
this.$refs.wrapper.addEventListener('touchmove', this.handleTouchMove)
this.$refs.wrapper.addEventListener('touchend', this.handleTouchEnd)
},
beforeDestroy() {
this.$refs.wrapper.removeEventListener('touchstart', this.handleTouchStart)
this.$refs.wrapper.removeEventListener('touchmove', this.handleTouchMove)
this.$refs.wrapper.removeEventListener('touchend', this.handleTouchEnd)
},
methods: {
handleTouchStart(e) {
if (this.$refs.wrapper.scrollTop === 0) {
this.startY = e.touches[0].clientY
this.isDragging = true
}
},
handleTouchMove(e) {
if (!this.isDragging) return
this.moveY = e.touches[0].clientY
this.distance = this.moveY - this.startY
if (this.distance > 0) {
e.preventDefault()
this.$refs.wrapper.style.transform = `translate3d(0, ${this.distance}px, 0)`
}
},
handleTouchEnd() {
if (!this.isDragging) return
this.isDragging = false
if (this.distance >= 80) {
this.$emit('refresh')
} else {
this.$refs.wrapper.style.transform = `translate3d(0, 0, 0)`
}
this.distance = 0
}
}
}
</script>
<style scoped>
.pull-to-refresh {
position: relative;
overflow: auto;
-webkit-overflow-scrolling: touch;
}
.pull-to-refresh__icon {
font-size: 20px;
color: #999;
text-align: center;
margin-bottom: 10px;
}
.pull-to-refresh__text {
font-size: 14px;
color: #999;
text-align: center;
margin-bottom: 10px;
}
.pull-to-refresh__content {
position: relative;
z-index: 1;
transform: translate3d(0, 0, 0);
transition: all 0.3s ease-in-out;
}
</style>
```
这个组件会在用户下拉到一定距离后触发 `refresh` 事件,你可以在使用组件的地方监听该事件并执行对应的操作,例如重新加载数据。
```vue
<template>
<pull-to-refresh @refresh="loadData">
<ul>
<li v-for="item in items" :key="item.id">{{item.title}}</li>
</ul>
</pull-to-refresh>
</template>
<script>
import PullToRefresh from './PullToRefresh.vue'
export default {
components: {
PullToRefresh
},
data() {
return {
items: []
}
},
methods: {
loadData() {
// 加载数据的逻辑
this.items = [...]
}
}
}
</script>
```
阅读全文
相关推荐
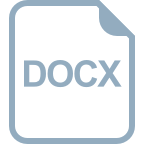

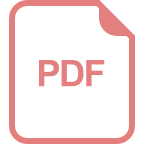
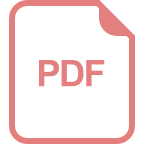
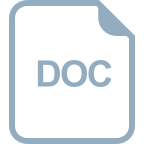
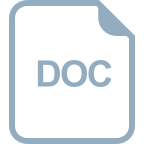
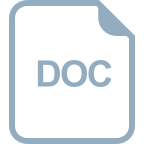
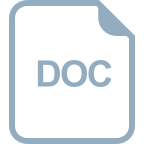


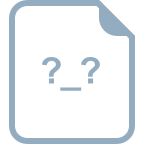
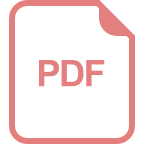
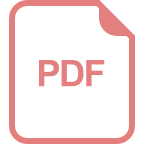
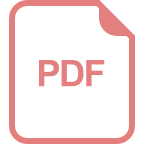